Create a text file and write text programmatically
The following asp.net c# example code demonstrates how we can create a
text file and write text on it programmatically in the .net framework.
We can get the application's physical path using HttpRequest.PhysicalApplicationPath property as Request.PhysicalApplicationPath. We also can get the file path by adding two strings, which are the physical application path and file name with extension. In this example, we created a text file name Text.txt and programmatically wrote two lines of text in this file at run time.
StreamWriter Class allows us to implement a TextWriter for writing characters to a stream in a particular encoding. In this example code, we initialize a StreamWriter object.
The File class’s CreateText(path) method creates or opens a file for writing UTF-8 encoded text. this method requires passing a parameter name 'path'. The path parameter value specifies the file to be opened for writing. This method’s return value type is System.IO.StreamWriter.
StreamWriter WriteLine(String) method allows us to write a string followed by a line terminator to the text string or stream.
StreamWriter Flush() method clears all buffers for the current writer and causes any buffered data to be written to the underlying stream.
StreamWriter Close() method closes the current StreamWriter object and the underlying stream.
In an app.net page, we need to include the System.IO namespace before working with files and directories.
We can get the application's physical path using HttpRequest.PhysicalApplicationPath property as Request.PhysicalApplicationPath. We also can get the file path by adding two strings, which are the physical application path and file name with extension. In this example, we created a text file name Text.txt and programmatically wrote two lines of text in this file at run time.
StreamWriter Class allows us to implement a TextWriter for writing characters to a stream in a particular encoding. In this example code, we initialize a StreamWriter object.
The File class’s CreateText(path) method creates or opens a file for writing UTF-8 encoded text. this method requires passing a parameter name 'path'. The path parameter value specifies the file to be opened for writing. This method’s return value type is System.IO.StreamWriter.
StreamWriter WriteLine(String) method allows us to write a string followed by a line terminator to the text string or stream.
StreamWriter Flush() method clears all buffers for the current writer and causes any buffered data to be written to the underlying stream.
StreamWriter Close() method closes the current StreamWriter object and the underlying stream.
In an app.net page, we need to include the System.IO namespace before working with files and directories.
StreamWriter.aspx
<%@ Page Language="C#" %>
<%@ Import Namespace="System.IO" %>
<!DOCTYPE html>
<script runat="server">
protected void Button1_Click(object sender, System.EventArgs e)
{
string appPath = Request.PhysicalApplicationPath;
string filePath = appPath + "Text.txt";
StreamWriter w;
w = File.CreateText(filePath);
w.WriteLine("This is a test line.");
w.WriteLine("This is another line.");
w.Flush();
w.Close();
Label1.Text = "File created and write successfully!<br />";
Label1.Text += filePath;
}
</script>
<html xmlns="http://www.w3.org/1999/xhtml">
<head id="Head1" runat="server">
<title>How to create text file and write text programmatically in asp.net</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<h2 style="color:Red">asp.net StreamWriter example:<br />Create File and Write In It</h2>
<asp:Label
ID="Label1"
runat="server"
Font-Size="Large"
ForeColor="SeaGreen"
Font-Bold="true"
Font-Italic="true"
>
</asp:Label>
<br /><br />
<asp:Button
ID="Button1"
runat="server"
OnClick="Button1_Click"
Font-Bold="true"
Text="Create File and Write Text"
ForeColor="SeaGreen"
/>
</div>
</form>
</body>
</html>
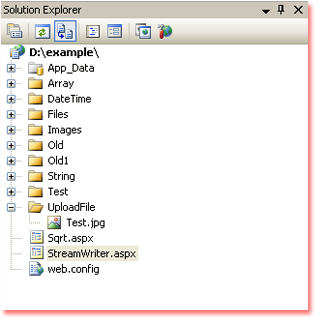
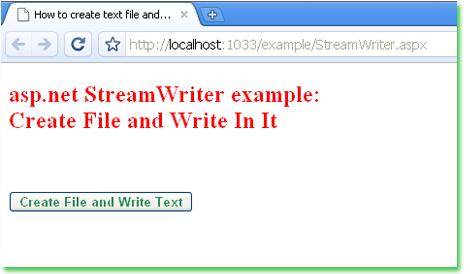
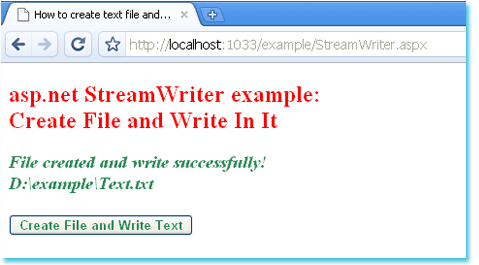
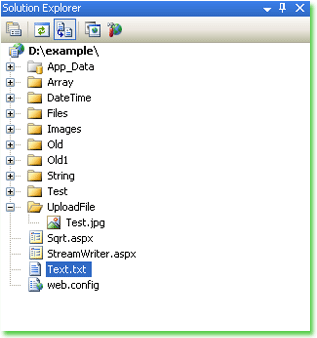
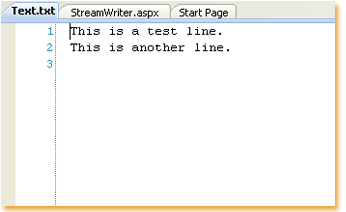