Introduction
ColdFusion is a powerful and easy-to-use platform for building dynamic web applications. One of its useful features is the ability to create and manage complex data structures. In this article, we will focus on creating structures (also known as associative arrays or dictionaries in other languages) using ColdFusion's StructNew
function. A structure is a collection of key-value pairs, making it an excellent tool for organizing related data, such as information about an employee.
This tutorial demonstrates a simple yet effective example of creating a structure to store employee information. We'll break down the components of the code and explain how ColdFusion allows developers to work efficiently with structures. Let’s dive into the example to better understand how the StructNew
function works in practice.
Creating the Structure with StructNew()
In ColdFusion, structures are created using the StructNew()
function. This function initializes a new, empty structure that can then be populated with key-value pairs. In our example, we start by creating a new structure named Employee
:
cfset Employee = StructNew()
The structure is essentially a container that can hold related data under specific keys. Once the structure is initialized, it is ready to store information.
Populating the Structure
After creating the structure, we assign values to it by adding key-value pairs. In this case, we store an employee’s first and last name within the structure:
cfset Employee.FirstName = "Jenny"
cfset Employee.LastName = "Jones"
Here, FirstName
and LastName
are the keys, and "Jenny" and "Jones" are their corresponding values. This demonstrates how ColdFusion allows you to easily group related information, making it convenient to manage multiple attributes for an object, such as an employee, within a single structure.
Displaying the Structure with cfdump
To visually inspect the contents of the structure, ColdFusion provides a handy tag called cfdump
. This tag outputs the structure in a human-readable format, making it easy to debug and verify the data stored within:
cfdump var="#Employee#" label="Employee"
In the browser, this will display a table showing the keys (FirstName
and LastName
) and their associated values ("Jenny" and "Jones"). This output helps ensure that the structure has been correctly initialized and populated.
HTML Integration
The example is wrapped within a simple HTML structure to ensure that the ColdFusion output is displayed properly in a web browser. The DOCTYPE
, head
, and body
tags are used to format the page, and the h2
tag is styled with inline CSS to give the title a pop of color (HotPink
). This demonstrates how ColdFusion code can be seamlessly integrated with HTML to build dynamic, data-driven web pages.
Summary
In this tutorial, we explored how to create and populate a structure in ColdFusion using the StructNew
function. Structures are a versatile way to organize related data, and ColdFusion makes it straightforward to manage them. We also learned how to output structure data using cfdump
, which is a useful tool for debugging.
By understanding how to create and manipulate structures, you can better manage complex data in your ColdFusion applications. This is just the beginning of what you can achieve with ColdFusion’s rich set of features for handling data structures.
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>StructNew function example: how to create a structure</title>
</head>
<body>
<h2 style="color:HotPink">StructNew Function Example</h2>
<cfset Employee=StructNew()>
<cfset Employee.FirstName="Jenny">
<cfset Employee.LastName="Jones">
<cfdump var="#Employee#" label="Employee">
</body>
</html>
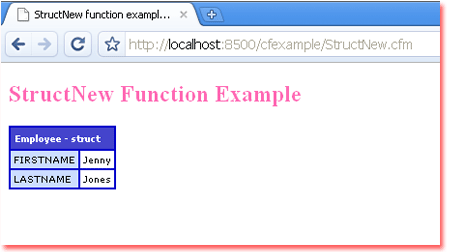
- IsStruct() - check whether a variable is a structure
- StructCount() - count the keys in a structure
- StructDelete() - remove an element from a structure
- StructIsEmpty() - determine whether a structure contains data
- StructKeyArray() - get an array of keys from a structure
- StructKeyExists() - check whether a specific key is present in a structure
- StructKeyList() - get a list of keys from a structure
- StructUpdate() - update a structure key with a value
- cfdump - dump a structure