Make Text Clickable In Compose
The Text widget is a very important widget of the android jetpack compose library. The Text widget allows us to display text objects inside its surface. Commonly Text widget displays simple text on it. But an android jetpack compose developer can style this text using the Text widget’s ‘style’ argument. This ‘style’ argument allows us to define text decoration, font family, font weight, text color, etc.
Android jetpack compose developer also can directly set The Text widget’s font family, font size, text color, text alignment, text letter spacing, etc using its parameters. But how can they set a click event for the Text widget when it has no default click event handler or onClick argument? The following code snippet will describe to us how we can set a click event handler for a Text widget.
The Text widget modifier object has an element name ‘clickable’. This ‘clickable()’ method allows us to set an onClick event to a Text widget. Modifier’s clickable() element has four arguments those are enabled, onClickLable, role and onClick. Those names are self-describable to their functionality. The ‘enabled’ augment allows us to enable and disable a Text widget onClick event. When we pass the true value to it then the Text widget is clickable and when we pass the false value then it is not clickable. True is its default value.
The ‘role’ argument is used for accessibility services. There are several types of Roles such as Button, Checkbox, Image, RadioButton, etc. The ‘onClick’ is the important argument here. This onClick parameter actually executes the click action. In this example code, we increment a number on the Text widget click event. On the Text click event, a click effect shows on the Text surface, so users can visualize that a click event occurred.
So finally, the Text widget modifier object’s ‘clickable’ element allows us to make a Text widget clickable. This kotlin code is written in an android studio IDE, copy the following code and paste it into your main activity kotlin file and run it on an emulator device to see the output. We also put a screenshot of this tutorial’s emulator screen.
Android jetpack compose developer also can directly set The Text widget’s font family, font size, text color, text alignment, text letter spacing, etc using its parameters. But how can they set a click event for the Text widget when it has no default click event handler or onClick argument? The following code snippet will describe to us how we can set a click event handler for a Text widget.
The Text widget modifier object has an element name ‘clickable’. This ‘clickable()’ method allows us to set an onClick event to a Text widget. Modifier’s clickable() element has four arguments those are enabled, onClickLable, role and onClick. Those names are self-describable to their functionality. The ‘enabled’ augment allows us to enable and disable a Text widget onClick event. When we pass the true value to it then the Text widget is clickable and when we pass the false value then it is not clickable. True is its default value.
The ‘role’ argument is used for accessibility services. There are several types of Roles such as Button, Checkbox, Image, RadioButton, etc. The ‘onClick’ is the important argument here. This onClick parameter actually executes the click action. In this example code, we increment a number on the Text widget click event. On the Text click event, a click effect shows on the Text surface, so users can visualize that a click event occurred.
So finally, the Text widget modifier object’s ‘clickable’ element allows us to make a Text widget clickable. This kotlin code is written in an android studio IDE, copy the following code and paste it into your main activity kotlin file and run it on an emulator device to see the output. We also put a screenshot of this tutorial’s emulator screen.
MainActivity.kt
package com.cfsuman.jetpackcompose
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import androidx.activity.compose.setContent
import androidx.compose.foundation.background
import androidx.compose.foundation.clickable
import androidx.compose.foundation.layout.*
import androidx.compose.foundation.shape.RoundedCornerShape
import androidx.compose.material.*
import androidx.compose.runtime.Composable
import androidx.compose.runtime.mutableStateOf
import androidx.compose.runtime.remember
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
import androidx.compose.ui.draw.clip
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.semantics.Role
import androidx.compose.ui.text.font.FontFamily
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.ui.unit.dp
import androidx.compose.ui.unit.sp
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
MainContent()
}
}
@Composable
fun MainContent(){
val txt = remember { mutableStateOf(0)}
Column(
modifier = Modifier
.fillMaxSize()
.background(Color(0xFFE5E4E2))
.padding(16.dp),
verticalArrangement = Arrangement.Center,
horizontalAlignment = Alignment.CenterHorizontally
) {
Text(
text = "${txt.value}",
fontFamily = FontFamily.Serif,
fontSize = 75.sp,
color = Color(0xFFE63E62),
modifier = Modifier
.clip(RoundedCornerShape(12.dp))
.background(Color(0xFFC9C0BB))
.clickable(
enabled = true,
role = Role.Button
){
txt.value += 1
}
.padding(25.dp)
)
}
}
@Preview
@Composable
fun ComposablePreview(){
//MainContent()
}
}
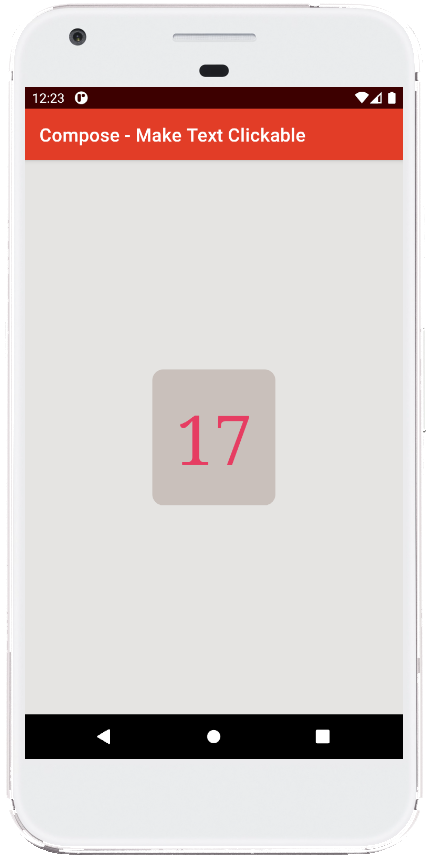
- jetpack compose - Infinite float animation
- jetpack compose - Animation start delay
- jetpack compose - Rotate animation
- jetpack compose - Draw line on Canvas
- jetpack compose - Button color
- jetpack compose - Button rounded corners
- jetpack compose - TextField clear focus
- jetpack compose - TextField focus change listener
- jetpack compose - Box rounded corners
- jetpack compose - Box center
- jetpack compose - Column center
- jetpack compose - Column background color
- jetpack compose - Column scrollable
- jetpack compose - Row spacing
- jetpack compose - Row scrolling