Selecting All Text on Focus in an EditText (Kotlin)
This code demonstrates two ways to achieve the same functionality in an Android app: selecting all the text inside an EditText when it receives focus. We'll explore both approaches, implemented in Kotlin and using an XML layout file.
Code Breakdown
The code consists of two parts: the MainActivity.kt
file and the activity_main.xml
layout file.
MainActivity.kt:
- Import Statements: We import necessary classes like
Activity
,Bundle
, andEditText
. - Class Definition: We define an
Activity
subclass namedMainActivity
. - Variable Declaration: An
EditText
variable namededitText2
is declared but not yet initialized. - onCreate Method: This method is called when the activity is first created.
- super.onCreate(savedInstanceState): This line calls the superclass's
onCreate
method. - setContentView(R.layout.activity_main): Sets the layout of the activity using the
activity_main.xml
file. - findViewById: We retrieve the
EditText
with the IDeditText2
from the layout and assign it to theeditText2
variable. - setSelectAllOnFocus(true): This line programmatically sets the behavior of
editText2
to select all the text when it gains focus.
- super.onCreate(savedInstanceState): This line calls the superclass's
activity_main.xml:
- Root Layout: We define a
ConstraintLayout
as the root layout of the activity. - First EditText (not used in this example): This
EditText
with IDeditText
is commented out in the provided code. It shows an alternative way to achieve the same functionality using XML attributes.- android:selectAllOnFocus="true": This attribute directly sets the behavior to select all text on focus for this specific
EditText
.
- android:selectAllOnFocus="true": This attribute directly sets the behavior to select all text on focus for this specific
- Second EditText (used in this example): This
EditText
with IDeditText2
is the one referenced in the Kotlin code.- Most attributes define its appearance and behavior (text size, input type, etc.).
Summary
The code provides two ways to make an EditText
select all its text when it receives focus. The first approach, implemented in MainActivity.kt
, uses the setSelectAllOnFocus(true)
method programmatically. The second approach, defined in activity_main.xml
, uses the android:selectAllOnFocus="true"
attribute within the XML layout file. Both methods achieve the same result: making the editing experience smoother by allowing users to quickly replace existing text when they tap on the EditText
.
package com.cfsuman.kotlintutorials
import android.app.Activity
import android.os.Bundle
import android.widget.EditText
class MainActivity : Activity() {
private lateinit var editText2:EditText
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
// get the widgets reference from XML layout
editText2 = findViewById(R.id.editText2)
// set edit text select all text on focus programmatically
editText2.setSelectAllOnFocus(true)
}
}
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#DCDCDC"
android:padding="32dp">
<!-- select all text inside edit text on focus in xml -->
<EditText
android:id="@+id/editText"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="12dp"
android:layout_marginEnd="12dp"
android:padding="12dp"
android:textSize="30sp"
android:inputType="text|textVisiblePassword"
android:fontFamily="sans-serif-condensed-medium"
android:selectAllOnFocus="true"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintVertical_bias="0.12" />
<EditText
android:id="@+id/editText2"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_marginStart="12dp"
android:layout_marginTop="24dp"
android:layout_marginEnd="12dp"
android:padding="12dp"
android:textSize="30sp"
android:inputType="text|textVisiblePassword"
android:fontFamily="sans-serif-condensed-medium"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/editText" />
</androidx.constraintlayout.widget.ConstraintLayout>
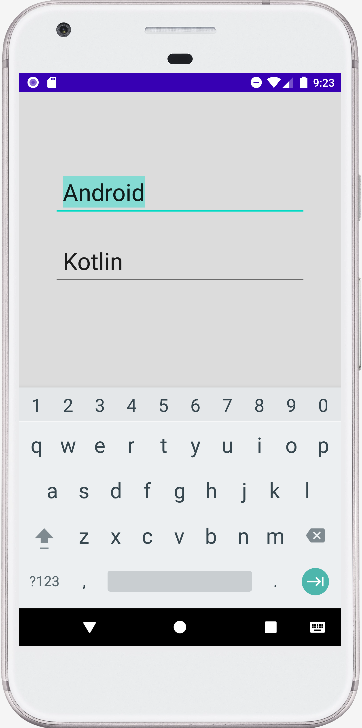
- android kotlin - EditText space validation
- android kotlin - EditText email validation
- android kotlin - EditText live characters count
- android kotlin - EditText first letter capitalization
- android kotlin - EditText allow only certain characters
- android kotlin - Set EditText digits programmatically
- android kotlin - EditText allow only positive numbers
- android kotlin - EditText allow only characters and numbers
- android kotlin - EditText numbers only programmatically
- android kotlin - EditText min length
- android kotlin - EditText set max length programmatically
- android kotlin - EditText rounded corners programmatically
- android kotlin - EditText border color programmatically
- android kotlin - EditText focus change listener
- android kotlin - EditText hide keyboard on lost focus