This code demonstrates how to adjust the transparency (alpha) of a Bitmap in an Android application written in Kotlin. It achieves this by creating a new Bitmap with a specific level of transparency and then displaying both the original and transparent versions in separate ImageViews.
The code includes three parts:
- MainActivity.kt: This is the main activity class that handles the UI and logic.
- assetsToBitmap: This is an extension function that retrieves a Bitmap from the app's assets folder.
- setAlpha: This is another extension function that creates a new Bitmap with a specific alpha value (transparency level).
Breakdown of the Code
MainActivity.kt
- The code first retrieves references to two ImageViews and two TextViews from the activity's layout using
findViewById
. - It then loads a bitmap from the assets folder using the
assetsToBitmap
extension function. - The
bitmap?.apply
block checks if the bitmap is loaded successfully and then performs the following actions:- Sets the original bitmap to the first ImageView and updates the corresponding TextView with a label.
- Calls the
setAlpha
function on the original bitmap with an alpha value of 75 (semi-transparent). - Sets the resulting transparent bitmap to the second ImageView and updates the corresponding TextView with a label and explanation of the alpha range (0-255).
assetsToBitmap
- This function takes a filename as input and attempts to open the corresponding file from the assets folder.
- If successful, it decodes the file into a Bitmap object and returns it.
- In case of any errors during the process, it prints the error message and returns null.
setAlpha
- This function takes a Bitmap object and an alpha value (between 0 and 255) as input.
- It creates a new Bitmap with the same dimensions and configuration (ARGB_8888) as the original one.
- It then creates a
Paint
object and sets its alpha value to the provided parameter. - Finally, it draws the original bitmap onto the new one using a
Canvas
object with the modified paint, effectively creating a copy with adjusted transparency.
Summary
This code snippet provides a clear and concise approach to adjusting the alpha of a Bitmap in Kotlin. It utilizes extension functions for cleaner code and offers informative descriptions within the TextView elements to enhance user understanding.
MainActivity.kt
package com.cfsuman.kotlintutorials
import android.app.Activity
import android.content.Context
import android.graphics.*
import android.os.Bundle
import android.widget.*
import java.io.IOException
class MainActivity : Activity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
// get the widgets reference from XML layout
val imageView = findViewById<ImageView>(R.id.imageView)
val imageView2 = findViewById<ImageView>(R.id.imageView2)
val textView = findViewById<TextView>(R.id.textView)
val textView2 = findViewById<TextView>(R.id.textView2)
// get the bitmap from assets folder
val bitmap = assetsToBitmap("flower103.jpg")
bitmap?.apply {
// show original bitmap in first image view
imageView.setImageBitmap(this)
textView.text = "Original Bitmap"
// show the transparent bitmap in image view
// int: set the alpha component [0..255]
// of the paint's color.
// 0 is full transparent
// 255 is full opaque
imageView2.setImageBitmap(setAlpha(75))
textView2.text = "Bitmap Alpha (75)"
textView2.append("\n\nRange 0..255")
textView2.append("\n0 is full transparent")
textView2.append("\n255 is full opaque")
}
}
}
// extension function to get bitmap from assets
fun Context.assetsToBitmap(fileName:String):Bitmap?{
return try {
val stream = assets.open(fileName)
BitmapFactory.decodeStream(stream)
} catch (e: IOException) {
e.printStackTrace()
null
}
}
// extension function to make transparent bitmap
fun Bitmap.setAlpha(alpha:Int):Bitmap?{
val bitmap = Bitmap.createBitmap(
width,height, Bitmap.Config.ARGB_8888
)
val paint = Paint()
// int: set the alpha component [0..255]
// of the paint's color.
// 0 is full transparent
// 255 is full opaque
paint.alpha = alpha
Canvas(bitmap).drawBitmap(
this,0f,0f,paint
)
return bitmap
}
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:id="@+id/rootLayout"
android:background="#DCDCDC"
android:padding="24dp">
<ImageView
android:id="@+id/imageView"
android:layout_width="0dp"
android:layout_height="250dp"
android:layout_marginTop="8dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="8dp"
android:text="Original Bitmap"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="@+id/imageView"
app:layout_constraintTop_toBottomOf="@+id/imageView" />
<ImageView
android:id="@+id/imageView2"
android:layout_width="0dp"
android:layout_height="250dp"
android:layout_marginTop="32dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView" />
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="8dp"
android:text="Transparent Bitmap"
app:layout_constraintEnd_toEndOf="@+id/imageView2"
app:layout_constraintStart_toStartOf="@+id/imageView2"
app:layout_constraintTop_toBottomOf="@+id/imageView2" />
</androidx.constraintlayout.widget.ConstraintLayout>
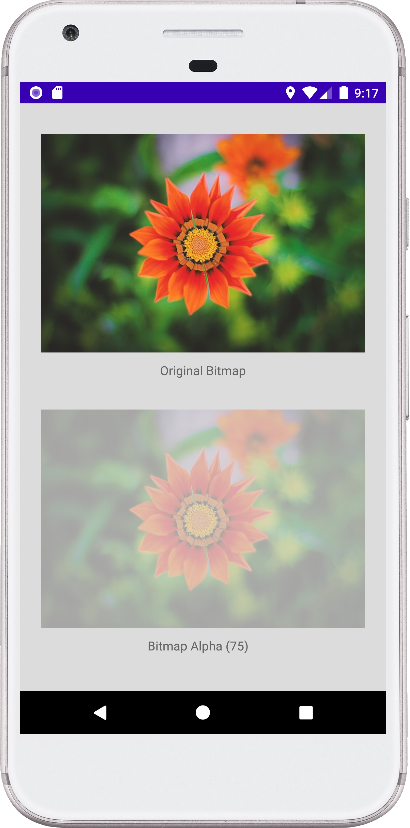
- android kotlin - BitmapShader example
- android kotlin - Bitmap tint solid color
- android kotlin - Bitmap mask
- android kotlin - Bitmap crop square
- android kotlin - Rounded corners bitmap
- android kotlin - Bitmap add padding
- android kotlin - Bitmap draw shadow
- android kotlin - Draw text on bitmap
- android kotlin - Bitmap color matrix set scale
- android kotlin - Bitmap sharpen
- android kotlin - Bitmap black and white effect
- android kotlin - Bitmap sepia effect
- android kotlin - Bitmap lighting color filter
- android kotlin - Bitmap porter duff color filter
- android kotlin - Bitmap change opacity