MainActivity.kt
package com.cfsuman.kotlintutorials
import android.os.Bundle
import android.widget.TextView
import androidx.appcompat.app.AppCompatActivity
import androidx.swiperefreshlayout.widget.SwipeRefreshLayout
import kotlin.random.Random
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
// Get the widgets reference from XML layout
val swipeRefreshLayout =
findViewById<SwipeRefreshLayout>(R.id.swipeRefreshLayout)
val textView = findViewById<TextView>(R.id.textView)
// Set an on refresh listener for swipe refresh layout
swipeRefreshLayout.setOnRefreshListener {
textView.text = "Refreshed & Generated Random Number\n" +
"${Random.nextInt(500)}"
// Hide swipe to refresh icon animation
swipeRefreshLayout.isRefreshing = false
}
}
}
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.swiperefreshlayout.widget.SwipeRefreshLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/swipeRefreshLayout"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="#F8F8F8"
android:padding="24dp">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Swipe to refresh me"
android:gravity="center"
android:textSize="28sp"
android:fontFamily="sans-serif"/>
</androidx.swiperefreshlayout.widget.SwipeRefreshLayout>
build.gradle [app] [dependencies]
implementation 'androidx.swiperefreshlayout:swiperefreshlayout:1.1.0'
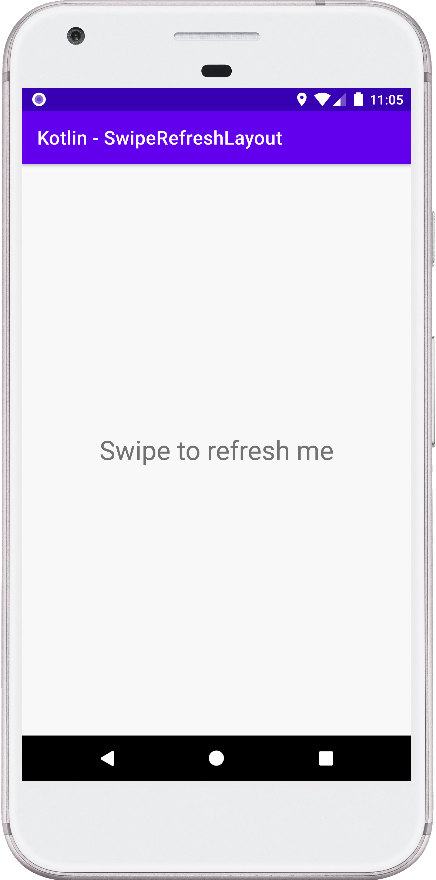

