Find the second occurrence of a substring within a String
The String represents text as a sequence of UTF-16 code units. The
String is a sequential collection of characters that is used to represent
text. The String is a sequential collection of System.Char objects.
The following .net c# tutorial code demonstrates how we can find the second occurrence of a substring within a String. In this .net c# tutorial code we will find that a specified substring is exist more than one time inside a String object and we will get the second occurrence index position of the substring.
Such as we have a String instance, we have to find a substring ‘Birch’ within it and we also have to show the index position of the ‘Birch’ substring’s second occurrence on the user interface. The substring maybe exists more than two times within the String instance but we have to find the second occurrence index position of the specified substring within the String instance.
We can do this using a ‘while’ loop. We will loop through all occurrences of the specified substring inside the String instance. When we get the second occurrence of the substring within the String instance, we will collect its index position and show it on the user interface. At that time we also break the loop because our desired result is found.
We can find the index position of the specified substring within a String instance using the String IndexOf() method. The String IndexOf() method reports the zero-based index of the first occurrence of a specified Unicode character or String within this instance. The IndexOf() method returns -1 if the character or String is not found in the instance.
The String IndexOf(string value, int startIndex) method overload reports the zero-based index of the first occurrence of the specified String in this instance and the search starts at a specified character position.
The following .net c# tutorial code demonstrates how we can find the second occurrence of a substring within a String. In this .net c# tutorial code we will find that a specified substring is exist more than one time inside a String object and we will get the second occurrence index position of the substring.
Such as we have a String instance, we have to find a substring ‘Birch’ within it and we also have to show the index position of the ‘Birch’ substring’s second occurrence on the user interface. The substring maybe exists more than two times within the String instance but we have to find the second occurrence index position of the specified substring within the String instance.
We can do this using a ‘while’ loop. We will loop through all occurrences of the specified substring inside the String instance. When we get the second occurrence of the substring within the String instance, we will collect its index position and show it on the user interface. At that time we also break the loop because our desired result is found.
We can find the index position of the specified substring within a String instance using the String IndexOf() method. The String IndexOf() method reports the zero-based index of the first occurrence of a specified Unicode character or String within this instance. The IndexOf() method returns -1 if the character or String is not found in the instance.
The String IndexOf(string value, int startIndex) method overload reports the zero-based index of the first occurrence of the specified String in this instance and the search starts at a specified character position.
string-find-second-occurrence.aspx
<%@ Page Language="C#" AutoEventWireup="true"%>
<!DOCTYPE html>
<script runat="server">
protected void Button1_Click(object sender, System.EventArgs e)
{
//this section create a string variable.
string plants = "Paper Birch. Red Birch. Bay Laurel. Silver Birch.";
Label1.Text = "string of plants..................<br />";
Label1.Text += plants+"<br />";
//substring to find/search indexes/occurrences in string.
string indexOfSubStringToFind = "Birch";
int index = 0;
int counter = 0;
//find all indexes/occurrences of specified substring in string
while ((index=plants.IndexOf(indexOfSubStringToFind, index)) != -1)
{
index++;
counter++;
//this line check second occurrence of search substring.
if (counter == 2)
{
Label1.Text += "<br />Second occurrence of [Birch] found at index: " + index;
break;
}
}
}
</script>
<html xmlns="http://www.w3.org/1999/xhtml">
<head id="Head1" runat="server">
<title>c# example - string find second occurrence</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<h2 style="color:MidnightBlue; font-style:italic;">
c# example - string find second occurrence
</h2>
<hr width="550" align="left" color="Gainsboro" />
<asp:Label
ID="Label1"
runat="server"
Font-Size="Large"
>
</asp:Label>
<br /><br />
<asp:Button
ID="Button1"
runat="server"
Text="string find second occurrence"
OnClick="Button1_Click"
Height="40"
Font-Bold="true"
/>
</div>
</form>
</body>
</html>
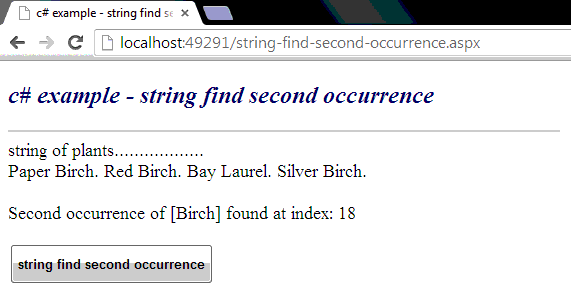