Find the index of a substring or character in a String
The String represents text as a sequence of UTF-16 code units. The
String is a sequential collection of characters that is used to represent
text. The String is a sequential collection of System.Char objects.
The following .net c# tutorial code demonstrates how we can find the index of a substring or a character in a String object. So, in this .net c# tutorial code we will find the index position of a specified substring or a specified character within a String instance. Here we will find the index of a substring or a character from a String object using the String IndexOf() method.
The String IndexOf() method reports the zero-based index of the first occurrence of a specified Unicode character or string within this instance. The String IndexOf() method returns -1 if the character or String is not found in this instance.
The String IndexOf(String) method overload reports the zero-based index of the first occurrence of the specified String in this instance. So using this method overload we can find a specified substring’s index position in a String instance. This String IndexOf(String) method returns the index position of the specified substring if it is found in the String instance otherwise it returns -1 if it is not found.
The String IndexOf(Char) method overload reports the zero-based index of the first occurrence of the specified Unicode character in this string. So using this method overload we can find the index position of a specified character from a String instance. This method overload returns the index position of the specified character if it is found in the String instance otherwise it returns -1 if it is not found in the String instance.
The following .net c# tutorial code demonstrates how we can find the index of a substring or a character in a String object. So, in this .net c# tutorial code we will find the index position of a specified substring or a specified character within a String instance. Here we will find the index of a substring or a character from a String object using the String IndexOf() method.
The String IndexOf() method reports the zero-based index of the first occurrence of a specified Unicode character or string within this instance. The String IndexOf() method returns -1 if the character or String is not found in this instance.
The String IndexOf(String) method overload reports the zero-based index of the first occurrence of the specified String in this instance. So using this method overload we can find a specified substring’s index position in a String instance. This String IndexOf(String) method returns the index position of the specified substring if it is found in the String instance otherwise it returns -1 if it is not found.
The String IndexOf(Char) method overload reports the zero-based index of the first occurrence of the specified Unicode character in this string. So using this method overload we can find the index position of a specified character from a String instance. This method overload returns the index position of the specified character if it is found in the String instance otherwise it returns -1 if it is not found in the String instance.
string-find-index.aspx
<%@ Page Language="C#" AutoEventWireup="true"%>
<!DOCTYPE html>
<script runat="server">
protected void Button1_Click(object sender, System.EventArgs e)
{
//this section create a string variable.
string plants = "Hard Thistle. Cutleaf Toothwort. Leatherleaf Viburnum";
Label1.Text = "string of plants..................<br />";
Label1.Text += plants+"<br />";
string indexToFind = "Cutleaf";
char indexToFind2 = 'T';
//this line find/search index of a substring in string
if (plants.IndexOf(indexToFind) != -1)
{
Label1.Text += "<br />Cutleaf found in string index of: ";
Label1.Text += plants.IndexOf(indexToFind);
}
else
{
Label1.Text += "<br />Cutleaf not found in string";
}
//this line find/search index of a character in string
if (plants.IndexOf(indexToFind2) != -1)
{
Label1.Text += "<br />character 'T' found in string index of: ";
Label1.Text += plants.IndexOf(indexToFind2);
}
else
{
Label1.Text += "<br />character 'T' not found in string";
}
}
</script>
<html xmlns="http://www.w3.org/1999/xhtml">
<head id="Head1" runat="server">
<title>c# example - string find index</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<h2 style="color:MidnightBlue; font-style:italic;">
c# example - string find index
</h2>
<hr width="550" align="left" color="Gainsboro" />
<asp:Label
ID="Label1"
runat="server"
Font-Size="Large"
>
</asp:Label>
<br /><br />
<asp:Button
ID="Button1"
runat="server"
Text="string find index"
OnClick="Button1_Click"
Height="40"
Font-Bold="true"
/>
</div>
</form>
</body>
</html>
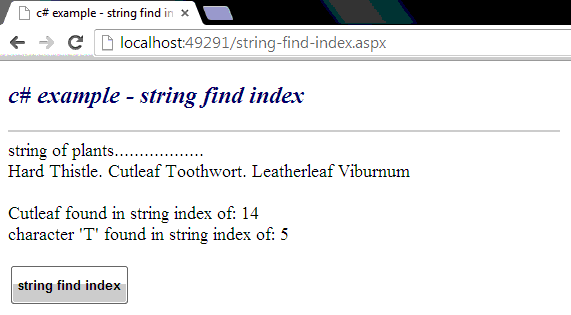