DataTable Compute() Method
.NET framework's DataTable represents one table of in-memory data. The
DataTable class exists in the System.Data namespace.
DataTable Compute() method allows us to compute the given expression on the current rows that pass the filter criteria. DataTable Compute() method requires passing two parameters named expression and filter.
Both parameters value data type are String. The expression parameter represents the expression to compute and the filter parameter represents the filter to limit the rows that evaluate in the expression.
DataTable Compute() method return value type is Object which represents an Object, set to the result of the computation. Compute() method return DBNull.Value, if the expression evaluates to null.
We can pass the expression parameter value as:
"Count(BookName)" where 'BookName' is a column name.
"Sum(Quantity * BookPrice)" where 'Quantity' and 'BookPrice' are column names.
We can pass the filter parameter value as "CategoryID = 5" where 'CategoryID' is a column name.
The following ADO.NET C# example code demonstrates to us how can we apply DataTable Compute method in an ASP.NET application.
DataTable Compute() method allows us to compute the given expression on the current rows that pass the filter criteria. DataTable Compute() method requires passing two parameters named expression and filter.
Both parameters value data type are String. The expression parameter represents the expression to compute and the filter parameter represents the filter to limit the rows that evaluate in the expression.
DataTable Compute() method return value type is Object which represents an Object, set to the result of the computation. Compute() method return DBNull.Value, if the expression evaluates to null.
We can pass the expression parameter value as:
"Count(BookName)" where 'BookName' is a column name.
"Sum(Quantity * BookPrice)" where 'Quantity' and 'BookPrice' are column names.
We can pass the filter parameter value as "CategoryID = 5" where 'CategoryID' is a column name.
The following ADO.NET C# example code demonstrates to us how can we apply DataTable Compute method in an ASP.NET application.
DataTableComputeMethod.aspx
<%@ Page Language="C#" AutoEventWireup="true" %>
<%@ Import Namespace="System.Data" %>
<!DOCTYPE html>
<script runat="server">
protected void Button1_Click(object sender, System.EventArgs e)
{
DataTable dt = new DataTable();
dt.TableName = "Books";
DataColumn dc1 = new DataColumn();
dc1.ColumnName = "CategoryID";
dc1.DataType = typeof(int);
dc1.AllowDBNull = false;
dc1.Unique = false;
DataColumn dc2 = new DataColumn();
dc2.ColumnName = "BookName";
dc2.DataType = typeof(string);
DataColumn dc3 = new DataColumn();
dc3.ColumnName = "Author";
dc3.DataType = typeof(string);
DataColumn dc4 = new DataColumn();
dc4.ColumnName = "Price";
dc4.DataType = typeof(decimal);
dt.Columns.AddRange(new DataColumn[] { dc1, dc2, dc3,dc4 });
dt.Rows.Add(new object[] { 1, "asp.net example ebook", "Jones",75 });
dt.Rows.Add(new object[] { 2, "coldfusion example ebook", "Jenny", 45 });
dt.Rows.Add(new object[] { 2, "flex example ebook", "Anne", 65 });
dt.AcceptChanges();
GridView1.DataSource = dt;
GridView1.DataBind();
string totalPrice = dt.Compute("Sum(Price)", "CategoryID = 2").ToString();
Label1.Text ="Total Price (CategoryID=2): " + totalPrice.ToString();
}
</script>
<html xmlns="http://www.w3.org/1999/xhtml">
<head id="Head1" runat="server">
<title>How to use DataTable Compute Method in ado.net</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<h2 style="color:DarkBlue; font-style:italic;">
How to use DataTable Compute Method in ado.net
</h2>
<hr width="525" align="left" color="CornFlowerBlue" />
<asp:GridView
ID="GridView1"
runat="server"
BorderColor="Snow"
ForeColor="Snow"
Width="525"
>
<HeaderStyle BackColor="OrangeRed" />
<RowStyle BackColor="Tan" />
<AlternatingRowStyle BackColor="BurlyWood" />
</asp:GridView>
<br />
<asp:Label
ID="Label1"
runat="server"
Font-Size="Large"
ForeColor="DodgerBlue"
Font-Italic="true"
>
</asp:Label>
<br /><br />
<asp:Button
ID="Button1"
runat="server"
OnClick="Button1_Click"
Text="Populate GridView"
Height="45"
Font-Bold="true"
ForeColor="DodgerBlue"
/>
</div>
</form>
</body>
</html>
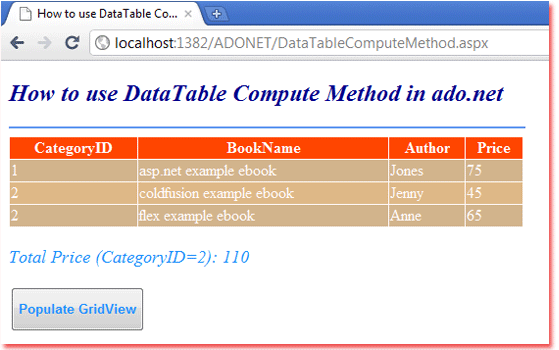