Get the source table of a DataView
The DataView is a customized view of a DataTable for sorting, filtering,
searching, editing, and navigation. The DataView does not store data. When the
asp.net developer changes the DataView's data that will affect the DataTable.
But when they change the DataTable's data that will affect all associated
DataViews. The DataView is much like a database view.
The following asp.net c# tutorial code demonstrates how we can get the source table of a DataView. Here we used the DataView class Table property to get the source DataTable object of a specified DataView instance.
The DataView class Table property gets or sets the source DataTable. The Table property value is a DataTable that provides the data for this view.
The DataTable also has a DefaultView property which returns the default DataView for the table. The asp.net developers can only set the Table property if the current value is null.
The DataTable class represents one table of in-memory data. The DataTable is a central object in the ADO.NET library. The DataSet and DataView objects use DataTable.
So finally, the asp.net c# developers can get the source table (DataTable) of a DataView instance by using the DataView class Table property.
The following asp.net c# tutorial code demonstrates how we can get the source table of a DataView. Here we used the DataView class Table property to get the source DataTable object of a specified DataView instance.
The DataView class Table property gets or sets the source DataTable. The Table property value is a DataTable that provides the data for this view.
The DataTable also has a DefaultView property which returns the default DataView for the table. The asp.net developers can only set the Table property if the current value is null.
The DataTable class represents one table of in-memory data. The DataTable is a central object in the ADO.NET library. The DataSet and DataView objects use DataTable.
So finally, the asp.net c# developers can get the source table (DataTable) of a DataView instance by using the DataView class Table property.
DataViewTableProperty.aspx
<%@ Page Language="C#" AutoEventWireup="true" %>
<%@ Import Namespace="System.Data" %>
<!DOCTYPE html>
<script runat="server">
protected void Button1_Click(object sender, System.EventArgs e)
{
DataTable dt = new DataTable();
dt.TableName = "Books";
DataColumn dc1 = new DataColumn();
dc1.ColumnName = "BookID";
dc1.DataType = typeof(int);
dc1.AllowDBNull = false;
dc1.Unique = true;
DataColumn dc2 = new DataColumn();
dc2.ColumnName = "Category";
dc2.DataType = typeof(string);
DataColumn dc3 = new DataColumn();
dc3.ColumnName = "BookName";
dc3.DataType = typeof(string);
DataColumn dc4 = new DataColumn();
dc4.ColumnName = "Author";
dc4.DataType = typeof(string);
dt.Columns.AddRange(new DataColumn[] { dc1, dc2, dc3, dc4 });
dt.Rows.Add(new object[] { 1, "Apple", "iPad 2 Pocket Guide, The", "Jeff Carlson" });
dt.Rows.Add(new object[] { 2, "Ajax", "Head First Ajax", "Rebecca M. Riordan" });
dt.Rows.Add(new object[] { 3, "Ajax", "Ajax: The Definitive Guide", "Anthony T. Holdener III" });
dt.AcceptChanges();
GridView1.DataSource = dt.DefaultView;
GridView1.DataBind();
//this line create a new DataView
DataView dView = new DataView(dt);
dView.RowFilter = "Category = 'Ajax'";
Label1.Text = "Here we create a new DataView with RowFilter expression (Category = 'Ajax')";
GridView2.DataSource = dView;
GridView2.DataBind();
DataTable dViewSourceTable = dView.Table;
Label2.Text = "Here we get the source DataTable of DataView" ;
GridView3.DataSource = dViewSourceTable;
GridView3.DataBind();
}
</script>
<html xmlns="http://www.w3.org/1999/xhtml">
<head id="Head1" runat="server">
<title>How to use DataView Table property to get the source DataTable in ado.net</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<h2 style="color:DarkBlue; font-style:italic;">
How to use DataView Table property
<br /> to get the source DataTable in ado.net
</h2>
<hr width="575" align="left" color="CornFlowerBlue" />
<asp:GridView
ID="GridView1"
runat="server"
BorderColor="Snow"
ForeColor="Snow"
Width="575"
Font-Names="Courier"
>
<HeaderStyle BackColor="OliveDrab" Height="30" />
<RowStyle BackColor="LightCoral" />
<AlternatingRowStyle BackColor="LightSalmon" />
</asp:GridView>
<br />
<asp:Label
ID="Label1"
runat="server"
Font-Size="Large"
ForeColor="DodgerBlue"
Font-Italic="true"
>
</asp:Label>
<br />
<asp:GridView
ID="GridView2"
runat="server"
BorderColor="Snow"
ForeColor="Snow"
Width="575"
Font-Names="Courier"
>
<HeaderStyle BackColor="OliveDrab" Height="30" />
<RowStyle BackColor="LightCoral" />
<AlternatingRowStyle BackColor="LightSalmon" />
</asp:GridView>
<br />
<asp:Label
ID="Label2"
runat="server"
Font-Size="Large"
ForeColor="DodgerBlue"
Font-Italic="true"
>
</asp:Label>
<br />
<asp:GridView
ID="GridView3"
runat="server"
BorderColor="Snow"
ForeColor="Snow"
Width="575"
Font-Names="Courier"
>
<HeaderStyle BackColor="OliveDrab" Height="30" />
<RowStyle BackColor="LightCoral" />
<AlternatingRowStyle BackColor="LightSalmon" />
</asp:GridView>
<br />
<asp:Button
ID="Button1"
runat="server"
OnClick="Button1_Click"
Text="Populate GridView"
Height="45"
Font-Bold="true"
ForeColor="DodgerBlue"
/>
</div>
</form>
</body>
</html>
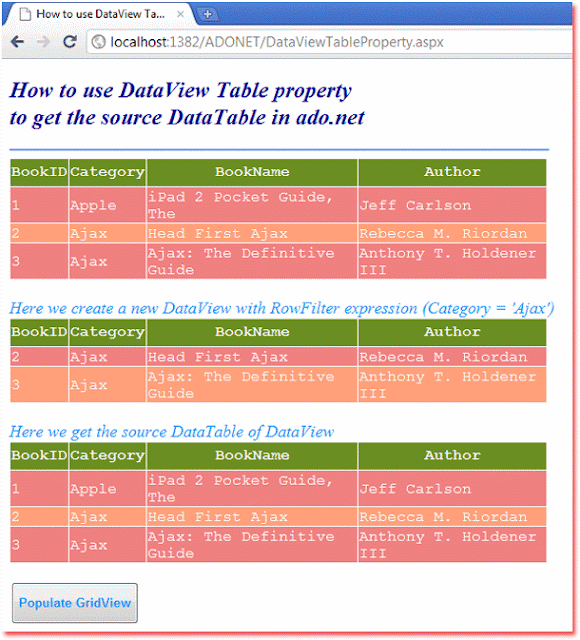