Count rows in a DataTable
The DataTable class represents one table of in-memory data. The
DataTable objects are conditionally case-sensitive. To create a DataTable
programmatically the asp.net developers must first define its schema by adding
DataColumn objects to the DataColumnCollection. To add rows to a DataTable,
the developers must use the NewRow() method to return a new DataRow object at
first. The DataTable also contains a collection of Constraint objects that can
be used to ensure the integrity of the data.
The following asp.net c# tutorial code demonstrates how we can count rows in a DataTable. So we have to count the DataRow objects within a DataTable instance. Here we used the DataTable class Rows property to get its rows collection as a DataRowCollection object. Then we used DataRowCollection’s Count property to count the rows within the rows collections.
The DataTable class Rows property gets the collection of rows that belong to this table. The Rows property value is a DataRowCollection that contains DataRow objects. Each DataRow in the collection represents a row of data in the table.
The DataRowCollection class represents a collection of rows for a DataTable. The DataRowCollection Count property gets the total number of DataRow objects in this collection. The Count property returns an Int32, the total number of DataRow objects in this collection.
So finally, using the DataTable class Rows property the asp.net web developers can get the DataRowCollection object. And the DataRowCollection class Count property allows the web developers to count the rows within the specified DataTable.
The following asp.net c# tutorial code demonstrates how we can count rows in a DataTable. So we have to count the DataRow objects within a DataTable instance. Here we used the DataTable class Rows property to get its rows collection as a DataRowCollection object. Then we used DataRowCollection’s Count property to count the rows within the rows collections.
The DataTable class Rows property gets the collection of rows that belong to this table. The Rows property value is a DataRowCollection that contains DataRow objects. Each DataRow in the collection represents a row of data in the table.
The DataRowCollection class represents a collection of rows for a DataTable. The DataRowCollection Count property gets the total number of DataRow objects in this collection. The Count property returns an Int32, the total number of DataRow objects in this collection.
So finally, using the DataTable class Rows property the asp.net web developers can get the DataRowCollection object. And the DataRowCollection class Count property allows the web developers to count the rows within the specified DataTable.
CountDataRowObjectsFromDataTable.aspx
<%@ Page Language="C#" AutoEventWireup="true" %>
<%@ Import Namespace="System.Data" %>
<!DOCTYPE html>
<script runat="server">
void Button1_Click(object sender, System.EventArgs e)
{
DataTable dt = new DataTable();
dt.TableName = "Books";
DataColumn dc1 = new DataColumn();
dc1.ColumnName = "BookID";
dc1.DataType = typeof(int);
dc1.AllowDBNull = false;
dc1.Unique = true;
DataColumn dc2 = new DataColumn();
dc2.ColumnName = "BookName";
dc2.DataType = typeof(string);
DataColumn dc3 = new DataColumn();
dc3.ColumnName = "Author";
dc3.DataType = typeof(string);
dt.Columns.AddRange(new DataColumn[] { dc1,dc2,dc3 });
dt.Rows.Add(new object[] { 1, "Oracle DBA Pocket Guide", "David C. Kreines" });
dt.Rows.Add(new object[] { 2, "Oracle SQL*Plus: The Definitive Guide, Second Edition", "Jonathan Gennick" });
dt.Rows.Add(new object[] { 3, "Oracle Utilities Pocket Reference", "Sanjay Mishra" });
GridView1.DataSource = dt;
GridView1.DataBind();
//this line count all DataRow from DataTable
int totalRows = dt.Rows.Count;
Label1.Text = "DataTable contains total DataRow objects: " + totalRows;
}
</script>
<html xmlns="http://www.w3.org/1999/xhtml">
<head id="Head1" runat="server">
<title>How to count total DataRow objects from a DataTable in ado.net</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<h2 style="color:DarkBlue; font-style:italic;">
How to count total DataRow
<br /> objects from a DataTable in ado.net
</h2>
<hr width="350" align="left" color="CornFlowerBlue" />
<asp:GridView
ID="GridView1"
runat="server"
BorderColor="Snow"
ForeColor="Snow"
Width="550"
>
<HeaderStyle BackColor="SaddleBrown" Height="35" />
<RowStyle BackColor="Purple" />
<AlternatingRowStyle BackColor="PaleVioletRed" />
</asp:GridView>
<br />
<asp:Label
ID="Label1"
runat="server"
Font-Size="X-Large"
ForeColor="OrangeRed"
Font-Italic="true"
>
</asp:Label>
<br /><br />
<asp:Button
ID="Button1"
runat="server"
OnClick="Button1_Click"
Text="Populate GridView"
Height="45"
Font-Bold="true"
ForeColor="DarkBlue"
/>
</div>
</form>
</body>
</html>
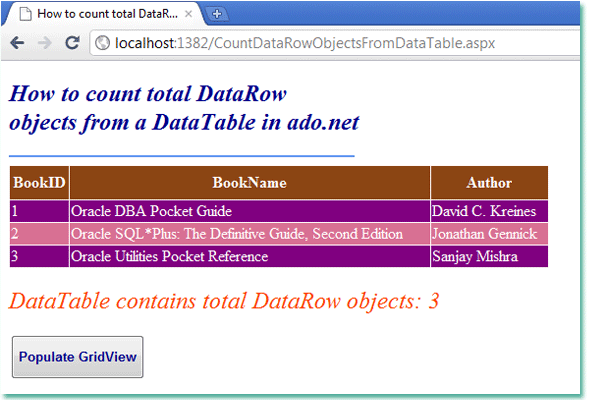