RegularExpressionValidator to validate US Phone Number
The RegularExpressionValidator control validates whether the value of an
associated input control matches the pattern specified by a regular
expression.
The following asp.net c# tutorial code demonstrates how we can validate a US Phone Number. In the below example, we validated a United States Phone Number by using a RegularExpressionValidator control.
The RegularExpressionValidator control checks whether the value of an input control (such as a TextBox) matches a pattern defined by a regular expression. Regular expression validation allows the asp.net c# developers to check for predictable sequences of characters, such as those in Zip Codes, telephone numbers, and URLs.
When the input control (such as a TextBox) is empty, the regular expression validation will succeed. So, if a value is required for the associated input control (such as a TextBox), the asp.net c# developers have to use a RequiredFieldValidator in addition to the RegularExpressionValidator web server control.
The RegularExpressionValidator ValidationExpression property allows the asp.net developers to get or set the regular expression that determines the pattern used to validate an input field (such as a TextBox). The ValidationExpression property value is a String that specifies the regular expression used to validate an input field for format. The default value of this property is Empty. The ValidationExpression property throws HttpException if the regular expression is not properly formed.
So finally, with a RegularExpressionValidator control, the asp.net c# developers can validate a US Phone Number. The developers just have to pass the specified regular expression to the ValidationExpression property value. The asp.net developers also have to use a RequiredFieldValidator control to make the input control (such as a TextBox) required.
The following asp.net c# tutorial code demonstrates how we can validate a US Phone Number. In the below example, we validated a United States Phone Number by using a RegularExpressionValidator control.
The RegularExpressionValidator control checks whether the value of an input control (such as a TextBox) matches a pattern defined by a regular expression. Regular expression validation allows the asp.net c# developers to check for predictable sequences of characters, such as those in Zip Codes, telephone numbers, and URLs.
When the input control (such as a TextBox) is empty, the regular expression validation will succeed. So, if a value is required for the associated input control (such as a TextBox), the asp.net c# developers have to use a RequiredFieldValidator in addition to the RegularExpressionValidator web server control.
The RegularExpressionValidator ValidationExpression property allows the asp.net developers to get or set the regular expression that determines the pattern used to validate an input field (such as a TextBox). The ValidationExpression property value is a String that specifies the regular expression used to validate an input field for format. The default value of this property is Empty. The ValidationExpression property throws HttpException if the regular expression is not properly formed.
So finally, with a RegularExpressionValidator control, the asp.net c# developers can validate a US Phone Number. The developers just have to pass the specified regular expression to the ValidationExpression property value. The asp.net developers also have to use a RequiredFieldValidator control to make the input control (such as a TextBox) required.
ValidateUSPhoneNumber.aspx
<%@ Page Language="C#" %>
<!DOCTYPE html>
<script runat="server">
protected void Page_Load(object sender, System.EventArgs e)
{
if(!this.IsPostBack)
{
Label1.Font.Bold = true;
Label1.Font.Italic = true;
Label1.Font.Size = FontUnit.Large;
Label1.ForeColor = System.Drawing.Color.SeaGreen;
TextBox1.BackColor = System.Drawing.Color.Tomato;
TextBox1.ForeColor = System.Drawing.Color.Snow;
}
}
protected void Button1_Click(object sender, EventArgs e)
{
Label1.Text = "Your Phone Number: " + TextBox1.Text.ToString();
}
</script>
<html xmlns="http://www.w3.org/1999/xhtml">
<head id="Head1" runat="server">
<title>asp.net RegularExpressionValidator example: how to validate U.S. Phone Number</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<h2 style="color:Navy">RegularExpressionValidator: U.S. Phone Number</h2>
<asp:Label
ID="Label1"
runat="server"
>
</asp:Label>
<br /><br />
<asp:Label
ID="Label2"
runat="server"
Text="U.S. Phone Number"
Font-Bold="true"
ForeColor="DodgerBlue"
>
</asp:Label>
<asp:TextBox
ID="TextBox1"
runat="server"
>
</asp:TextBox>
<asp:RequiredFieldValidator
ID="RequiredFieldValidator1"
runat="server"
ControlToValidate="TextBox1"
Text="*"
>
</asp:RequiredFieldValidator>
<asp:RegularExpressionValidator
ID="RegularExpressionValidator1"
runat="server"
ValidationExpression="((\(\d{3}\) ?)|(\d{3}-))?\d{3}-\d{4}"
ControlToValidate="TextBox1"
ErrorMessage="Input valid U.S. Phone Number!"
></asp:RegularExpressionValidator>
<br /><br />
<asp:Button
ID="Button1"
runat="server"
Text="Submit Phone Number"
Font-Bold="true"
ForeColor="DodgerBlue"
OnClick="Button1_Click"
/>
</div>
</form>
</body>
</html>

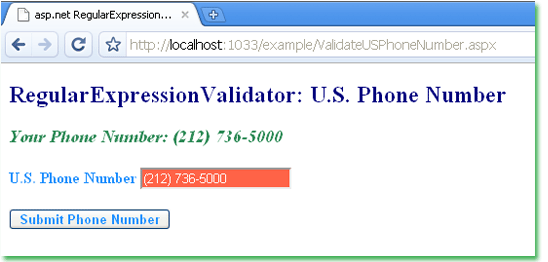