Introduction
The <cftable>
tag in Adobe ColdFusion provides a straightforward way to create HTML tables dynamically based on query results. This example demonstrates how to use <cftable>
and <cfcol>
tags in ColdFusion to generate an HTML table displaying author information from a database. This approach can be incredibly useful for web applications needing to display database-driven content in a structured and readable format. The example also includes configurations like column headers, borders, and maximum rows, showcasing how ColdFusion makes building HTML tables easy and efficient.
This tutorial will break down each section of the code, explaining how each part contributes to constructing an HTML table. We’ll cover the <cfquery>
tag, the <cftable>
tag with its various attributes, and the <cfcol>
tag for defining individual columns. Finally, we’ll summarize the example's utility and how it can be adapted to different datasets or design requirements.
Setting Up the Query with <cfquery>
The first step in this example is retrieving data from a database. Here, the <cfquery>
tag is used to create a query named qAuthors
, which retrieves the AuthorID
, FirstName
, and LastName
columns from the AUTHORS
table in the cfbookclub
data source. The query results will later be used by the <cftable>
tag to populate the HTML table with rows of author information.
The <cfquery>
tag connects to the specified data source and executes the SQL command provided, which makes it a fundamental part of any ColdFusion-based data-driven page. Once the query runs, the resulting data set is stored in the qAuthors
variable, making it available for use within the <cftable>
tag.
Constructing the Table with <cftable>
The main feature of this example is the <cftable>
tag, which creates the HTML table structure. The query
attribute specifies the data source for the table, which in this case is the qAuthors
query from earlier. By setting htmltable="yes"
, the table is rendered as an HTML table in the browser, enabling customization and easy viewing.
Several attributes control the appearance and functionality of the table. Setting border="yes"
adds visible borders around the table cells, and colheaders="yes"
ensures that column headers are included at the top of the table. The maxrows="8"
and startrow="1"
attributes limit the number of rows displayed and set the starting row, allowing for pagination if needed. Finally, colspacing="1"
and headerlines="1"
control the spacing between columns and the number of header lines, respectively, enhancing the table's readability.
Defining Columns with <cfcol>
Inside the <cftable>
tag, individual columns are defined using the <cfcol>
tag. Each <cfcol>
tag represents one column in the table, with attributes specifying column headers, alignment, and the data to display. Here, three <cfcol>
tags define columns for "Author ID," "First Name," and "Last Name," which display the values from the corresponding fields in the qAuthors
query.
The header
attribute in each <cfcol>
tag specifies the column name, such as "Author ID" for the first column. The align
attribute aligns text to the left, and the text
attribute defines what data to display, referencing the query fields (#AuthorID#
, #FirstName#
, and #LastName#
). This approach allows you to tailor each column individually, making it easy to manage how data appears in each column.
Summary
This example demonstrates how ColdFusion's <cftable>
and <cfcol>
tags make it simple to build HTML tables dynamically based on query results. By using these tags, you can create customizable, database-driven tables with minimal code, perfect for displaying information in a web-friendly format. The attributes in <cftable>
and <cfcol>
provide a range of options for styling and organizing data, allowing developers to tailor tables to their specific needs.
Whether you’re working with small datasets or large records, ColdFusion’s table-building capabilities can streamline your code and improve your workflow, making it a valuable tool for any web developer working with ColdFusion.
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>cftable tag example: how to build a table in coldfusion (HTML Table)</title>
</head>
<body>
<h2 style="color:Crimson">cftable Tag Example: HTML Table</h2>
<cfquery name="qAuthors" datasource="cfbookclub">
SELECT AuthorID, FirstName, LastName FROM AUTHORS
</cfquery>
<cftable
query="qAuthors"
border="yes"
maxrows="8"
startrow="1"
colspacing="1"
colheaders="yes"
htmltable="yes"
headerlines="1"
>
<cfcol header="Author ID" align="left" text="#AuthorID#">
<cfcol header="First Name" align="left" text="#FirstName#">
<cfcol header="Last Name" align="left" text="#LastName#">
</cftable>
</body>
</html>
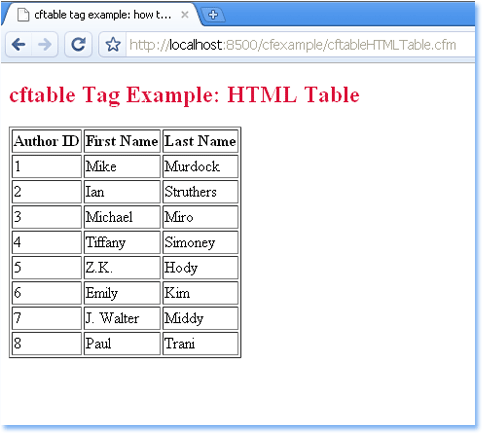