CompareValidator
CompareValidator control compares user inputted value with another input
control value or a constant value. CompareValidator validation success if the
inputted value matches the criteria specified by the Operator, ValueToCompare,
or ControlToCompare property.
CompareValidator Type property indicates whether inputted value needs to be converted to a specified data type (string, date, currency, double, or integer). The controlToValidate property indicates which control needs to validate. ControlToCompare property indicates which control needs to compare with inputted value (such as TextBox value).
ValueToCompare property indicates that you want to compare the inputted value with a constant value instead another control value. Don't put ControlToValidate and ValueToCompare properties together in a CompareValidator control. You can use any one property at a time. That means you can only compare with a control or compare with a constant value at the same time.
You can use the Operator property to specify the type of comparison to perform, such as equal to, greater than, equal, not equal, less than, less than equal, data type check, etc. Operator property value DataTypeCheck validated the data type specified by the Type property. so the CompareValidator validation fails if the inputted value cannot be converted to the specified data type.
This example demonstrates how can we compare (validate) to the password field (TextBox) using the ASP.NET CompareValidator control. If the user-inputted password does not match in both TextBoxes then the validation fails and the browser shows a validation error message. If both TextBox text matches then validation success.
CompareValidator Type property indicates whether inputted value needs to be converted to a specified data type (string, date, currency, double, or integer). The controlToValidate property indicates which control needs to validate. ControlToCompare property indicates which control needs to compare with inputted value (such as TextBox value).
ValueToCompare property indicates that you want to compare the inputted value with a constant value instead another control value. Don't put ControlToValidate and ValueToCompare properties together in a CompareValidator control. You can use any one property at a time. That means you can only compare with a control or compare with a constant value at the same time.
You can use the Operator property to specify the type of comparison to perform, such as equal to, greater than, equal, not equal, less than, less than equal, data type check, etc. Operator property value DataTypeCheck validated the data type specified by the Type property. so the CompareValidator validation fails if the inputted value cannot be converted to the specified data type.
This example demonstrates how can we compare (validate) to the password field (TextBox) using the ASP.NET CompareValidator control. If the user-inputted password does not match in both TextBoxes then the validation fails and the browser shows a validation error message. If both TextBox text matches then validation success.
CompareValidatorHowToUse.aspx
<%@ Page Language="C#" %>
<!DOCTYPE html>
<script runat="server">
protected void Button1_Click(object sender,System.EventArgs e){
Label1.Text = "Form Submited and Password match.";
}
</script>
<html xmlns="http://www.w3.org/1999/xhtml">
<head id="Head1" runat="server">
<title>CompareValidator example: how to use CompareValidator control in asp.net</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<asp:Label ID="Label1" runat="server"></asp:Label>
<br />
<asp:Label ID="Label2" runat="server" Text="<u>P</u>assword" AccessKey="P" AssociatedControlID="TextBox1"></asp:Label>
<asp:TextBox ID="TextBox1" runat="server" TextMode="Password"></asp:TextBox>
<asp:RequiredFieldValidator ID="RequiredFieldValidator1" runat="server" ControlToValidate="TextBox1" Text="*"></asp:RequiredFieldValidator>
<asp:CompareValidator ID="CompareValidator" runat="server" ControlToValidate="TextBox1" ControlToCompare="TextBox2" ErrorMessage="Password does not match!">
</asp:CompareValidator>
<br />
<asp:Label ID="Label3" runat="server" Text="Re-Type Password" AssociatedControlID="TextBox2"></asp:Label>
<asp:TextBox ID="TextBox2" runat="server" TextMode="Password"></asp:TextBox>
<br />
<asp:Button ID="Button1" runat="server" Text="Compare" OnClick="Button1_Click" />
</div>
</form>
</body>
</html>


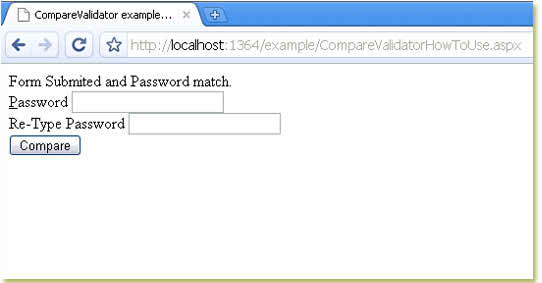