MainActivity.kt
package com.cfsuman.jetpackcompose
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import androidx.activity.compose.setContent
import androidx.compose.foundation.layout.*
import androidx.compose.material.*
import androidx.compose.runtime.*
import androidx.compose.ui.graphics.Color
import androidx.compose.ui.tooling.preview.Preview
import androidx.compose.material.Text
import androidx.compose.material.TopAppBar
import androidx.compose.material.icons.Icons
import androidx.compose.material.icons.filled.*
import androidx.compose.ui.Alignment
import androidx.compose.ui.Modifier
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContent {
GetScaffold()
}
}
@Composable
fun GetScaffold(){
val counter:MutableState<Int> = remember{ mutableStateOf(0)}
Scaffold(
topBar = { TopAppBarContent(counter) },
content = {MainContent(counter)},
backgroundColor = Color(0xFFEDEAE0)
)
}
@Composable
fun TopAppBarContent(counter: MutableState<Int>) {
TopAppBar(
title = { Text(text = "Compose - TopAppBar Actions")},
backgroundColor = Color(0xFFC0E8D5),
actions = {
IconButton(onClick = {
counter.value++;
}) {
Icon(
Icons.Filled.AddCircle,
contentDescription = "Localized description"
)
}
IconButton(onClick = {
counter.value--;
}) {
Icon(
Icons.Filled.Close,
contentDescription = "Localized description"
)
}
}
)
}
@Composable
fun MainContent(counter: MutableState<Int>){
Box(
modifier = Modifier.fillMaxSize(),
contentAlignment = Alignment.Center
){
Text(
text = "${counter.value}",
style = MaterialTheme.typography.h1
)
}
}
@Preview
@Composable
fun ComposablePreview(){
//GetScaffold()
}
}

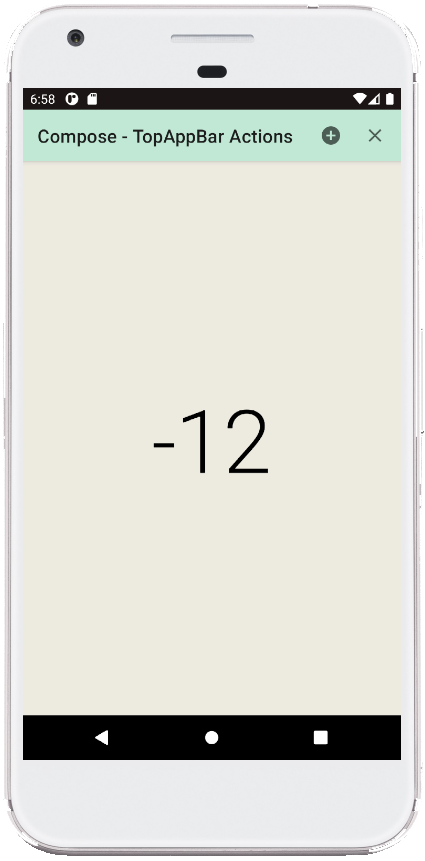
- jetpack compose - Row onClick
- jetpack compose - Row weight
- jetpack compose - Column weight
- jetpack compose - Row gravity
- jetpack compose - Row background
- jetpack compose - Column align bottom
- jetpack compose - Box center alignment
- jetpack compose - Box gravity
- jetpack compose - Card center
- jetpack compose - Card padding
- jetpack compose - Card background color
- jetpack compose - How to use ModalDrawer
- jetpack compose - How to use BadgeBox
- jetpack compose - Update state of another function variable
- jetpack compose - TopAppBar navigation