Centering the ActionBar Title in Android (Kotlin)
This code demonstrates how to center the title text displayed within the ActionBar of an Android application written in Kotlin. The default behavior of the ActionBar is to align the title text to the left side. This approach utilizes a custom view to achieve the desired centered alignment.
Code Breakdown
The MainActivity.kt
file defines the core logic for customizing the ActionBar title. Here's a breakdown of the key functionalities:
Setting Up the Custom Title:
- Inside
onCreate()
, thesupportActionBar?.apply
block handles modifications to the ActionBar. customView = actionBarCustomTitle()
assigns a custom TextView (created by theactionBarCustomTitle
function) as the ActionBar's custom view.displayOptions = DISPLAY_SHOW_CUSTOM
hides the default title and displays the custom view instead.- Additional lines set the app icon and enable its display.
- Inside
Creating the Custom Title View:
- The
actionBarCustomTitle
function creates a new TextView programmatically. - It sets the desired title text, creates layout parameters, and applies them to the TextView.
gravity = Gravity.CENTER
aligns the text within the TextView to the center.setTextAppearance
sets the visual style of the text, mimicking the default ActionBar title appearance.- Finally,
setTextColor
sets the text color to white.
- The
Summary
By employing a custom TextView with centered text alignment and appropriate styling, this code effectively positions the ActionBar title in the center. This approach offers more control over the title's appearance compared to relying solely on the default theme. It's important to note that this method removes the default title functionality, so consider adding alternative mechanisms for navigation or actions if needed within the ActionBar.
package com.cfsuman.kotlintutorials
import android.graphics.Color
import android.os.Bundle
import android.view.Gravity
import android.widget.TextView
import androidx.appcompat.app.ActionBar.DISPLAY_SHOW_CUSTOM
import androidx.appcompat.app.AppCompatActivity
import androidx.constraintlayout.widget.ConstraintLayout.LayoutParams
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
supportActionBar?.apply {
// Show custom title in action bar
customView = actionBarCustomTitle()
displayOptions = DISPLAY_SHOW_CUSTOM
setDisplayShowHomeEnabled(true)
setDisplayUseLogoEnabled(true)
setLogo(R.drawable.ic_action_help)
}
}
// Function to create a custom action bar title
private fun actionBarCustomTitle():TextView{
return TextView(this).apply {
// Set the action bar title text
text = "ActionBar Center Title"
// Create a layout params instance
val params = LayoutParams(
LayoutParams.MATCH_PARENT,
LayoutParams.WRAP_CONTENT
)
// Apply the layout parameters for text view
layoutParams = params
// Center align the text view/ action bar title
gravity = Gravity.CENTER
// Set the text appearance of action bar title text
setTextAppearance(
android.R.style
.TextAppearance_Material_Widget_ActionBar_Title
)
// Set the action bar title text color
setTextColor(Color.WHITE)
}
}
}
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:id="@+id/rootLayout"
android:background="#DCDCDC" />
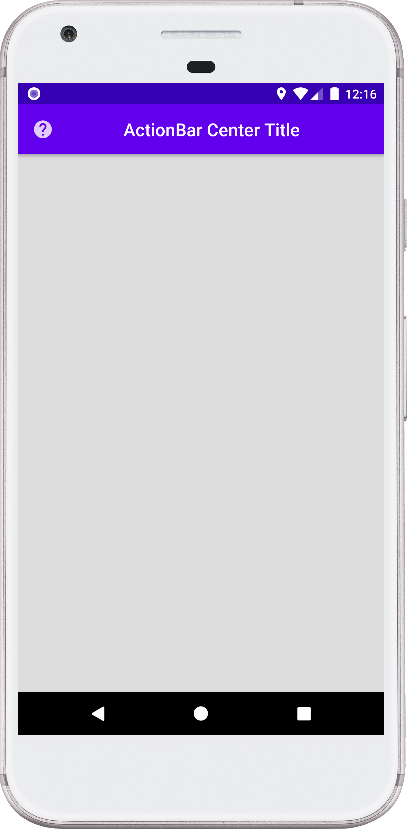