UWP ScrollViewer
The ScrollViewer class represents a scrollable area that can contain other visible elements. The following UWP app development tutorial code will demonstrate how we can use a ScrollViewer in a UWP application to enable the scrolling capability of the inside elements.
Here we put multiple TextBlock in a StackPanel control whose orientation is vertical. Then we wrap the StackPanel control with a ScrollViewer, so the users can scroll through the bottom of StackPanel content. The UWP developers use ScrollViewer container control to lets the user pan and zoom its content.
The ScrollViewer control enables content to be displayed in a smaller area than its actual size. The ScrollViewer displays scrollbars when its content is not entirely visible.
The ScrollViewer class’s HorizontalScrollBarVisibility property gets or sets a value that indicates whether a horizontal ScrollBar should be displayed in a ScrollViewer. This property value is a ScrollBarVisibility value that indicates whether a horizontal ScrollBar should be displayed. The default value of this property is Disabled. Here we used the value Auto which means a ScrollBar appears only when the viewport cannot display all of the content.
The ScrollViewer class VerticalScrollBarVisibility property gets or sets a value that indicates whether a vertical ScrollBar should be displayed. This property value is also a ScrollBarVisibility enum value that indicates whether a vertical ScrollBar should be displayed. The default value of this property is Visible. In this example code, we set this property value to Auto.
MainPage.xaml
<Page
x:Class="UniversalAppTutorials.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:UniversalAppTutorials"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d"
>
<ScrollViewer
Background="Orange"
HorizontalScrollBarVisibility="Auto"
VerticalScrollBarVisibility="Auto"
>
<!--
Use StackPanel Vertical orientation to enable
contents vertical scrolling. And use StackPanel
Horizontal orientation to enable contents horizontal scrolling.
-->
<StackPanel Orientation="Vertical" Padding="50">
<TextBlock Text="A" FontSize="100"/>
<TextBlock Text="B" FontSize="100"/>
<TextBlock Text="C" FontSize="100"/>
<TextBlock Text="D" FontSize="100"/>
<TextBlock Text="E" FontSize="100"/>
<TextBlock Text="F" FontSize="100"/>
<TextBlock Text="G" FontSize="100"/>
<TextBlock Text="H" FontSize="100"/>
<TextBlock Text="I" FontSize="100"/>
<TextBlock Text="J" FontSize="100"/>
<TextBlock Text="K" FontSize="100"/>
<TextBlock Text="L" FontSize="100"/>
<TextBlock Text="M" FontSize="100"/>
<TextBlock Text="N" FontSize="100"/>
<TextBlock Text="O" FontSize="100"/>
<TextBlock Text="P" FontSize="100"/>
<TextBlock Text="Q" FontSize="100"/>
<TextBlock Text="R" FontSize="100"/>
<TextBlock Text="S" FontSize="100"/>
<TextBlock Text="T" FontSize="100"/>
<TextBlock Text="U" FontSize="100"/>
<TextBlock Text="V" FontSize="100"/>
<TextBlock Text="W" FontSize="100"/>
<TextBlock Text="X" FontSize="100"/>
<TextBlock Text="Y" FontSize="100"/>
<TextBlock Text="Z" FontSize="100"/>
</StackPanel>
</ScrollViewer>
</Page>
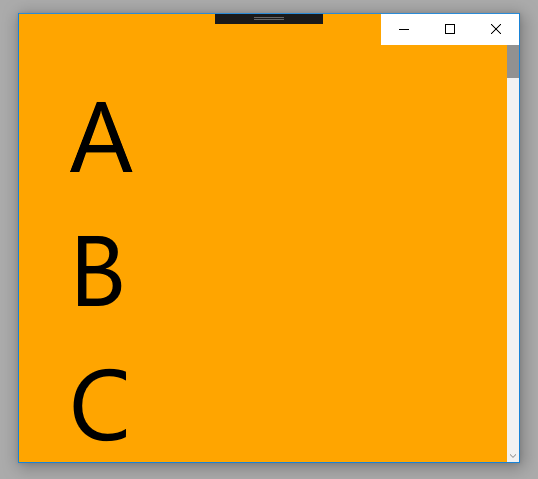
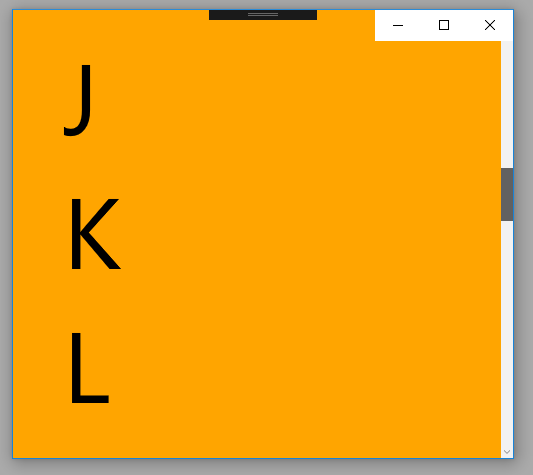
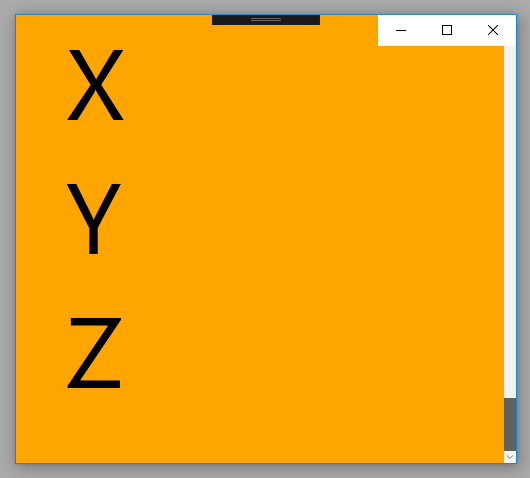