UWP - Get ComboBox Selected Value
The ComboBox class represents a selection control that combines a
non-editable text box and a drop-down list box that allows UWP app users to
select an item from a list.
The UWP app developers can use a ComboBox to present a list of items that the app user can select from. The ComboBox starts in a compact state and it expands to display a list of selectable items. The ComoboBox control is also known as a drop-down list.
The ComboBox either displays the current selection or is empty if there is no selected item in its closed state. ComboBox displays the list of selectable items in its expanded state.
The following UWP app development tutorial code demonstrates how we can get the ComboBox selected value. Here we data bind the ComboBox control with Dictionary items. We also add a selection changed event to the ComboBox control, so we can get the selected item value when the user makes a selection on the ComboBox. The Selector class’s SelectedValuePath property gets or sets the property path that is used to get the SelectedValue property of the SelectedItem property.
The Selector class’s SelectionChanged event occurs when the currently selected item changes. And the Selector class’s SelectedValue property gets or sets the value of the selected item, obtained by using the SelectedValuePath. So using the SelectionChanged event and SelectedValue property we can get the ComboBox selected value at runtime.
The UWP app developers can use a ComboBox to present a list of items that the app user can select from. The ComboBox starts in a compact state and it expands to display a list of selectable items. The ComoboBox control is also known as a drop-down list.
The ComboBox either displays the current selection or is empty if there is no selected item in its closed state. ComboBox displays the list of selectable items in its expanded state.
The following UWP app development tutorial code demonstrates how we can get the ComboBox selected value. Here we data bind the ComboBox control with Dictionary items. We also add a selection changed event to the ComboBox control, so we can get the selected item value when the user makes a selection on the ComboBox. The Selector class’s SelectedValuePath property gets or sets the property path that is used to get the SelectedValue property of the SelectedItem property.
The Selector class’s SelectionChanged event occurs when the currently selected item changes. And the Selector class’s SelectedValue property gets or sets the value of the selected item, obtained by using the SelectedValuePath. So using the SelectionChanged event and SelectedValue property we can get the ComboBox selected value at runtime.
MainPage.xaml
<Page
x:Class="UniversalAppTutorials.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:UniversalAppTutorials"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<StackPanel
x:Name="stack_panel1"
Orientation="Horizontal"
Background="Azure"
Padding="100"
>
<ComboBox
x:Name="ComboBox1"
Header="Select A Color"
SelectionChanged="ComboBox1_SelectionChanged"
>
</ComboBox>
<TextBlock
x:Name="TextBlock1"
FontFamily="Consolas"
FontSize="25"
Foreground="Navy"
Margin="50,5,5,5"
/>
</StackPanel>
</Page>
MainPage.xaml.cs
using Windows.UI.Xaml.Controls;
using System.Collections.Generic;
using System;
namespace UniversalAppTutorials
{
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
// Initialize a new empty Dictionary instance
Dictionary<String, string> colors = new Dictionary<string, string>();
// Put some key value pairs into the dictionary
colors.Add("SILVER", "#C0C0C0");
colors.Add("RED", "#FF0000");
colors.Add("GREEN", "#008000");
colors.Add("AQUA", "#00FFFF");
colors.Add("PURPLE", "#800080");
colors.Add("LIME", "#00FF00");
// Finally, Specify the ComboBox items source
ComboBox1.ItemsSource = colors;
// Specify the ComboBox items text and value
ComboBox1.SelectedValuePath = "Value";
ComboBox1.DisplayMemberPath = "Key";
}
private void ComboBox1_SelectionChanged(object sender, SelectionChangedEventArgs e)
{
// Get the ComboBox instance
ComboBox comboBox = sender as ComboBox;
// Get the ComboBox selected item value and display on TextBlock
TextBlock1.Text = "You selected:\n";
TextBlock1.Text += "Value : " + comboBox.SelectedValue.ToString();
}
}
}
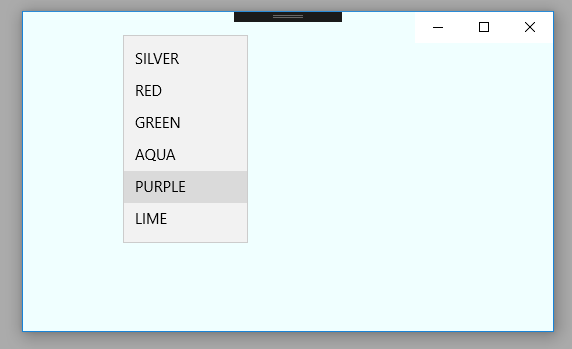
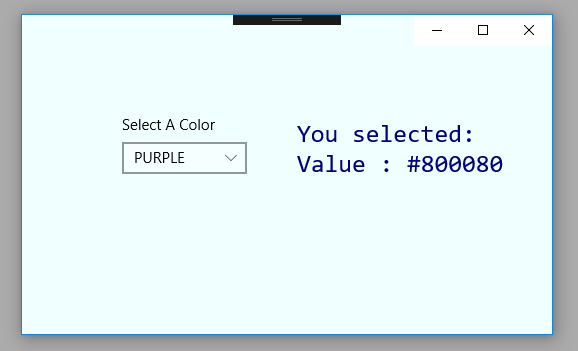