UWP - Get ComboBox Selected Item
The ComboBox class represents a selection control that combines a
non-editable text box and a drop-down list box that allows UWP app users to
select an item from a list. The ComboBox starts in a compact state and it
expands to display a list of selectable items. The ComoboBox control is also
known as a drop-down list.
The following UWP app development tutorial code demonstrates how we can get the ComboBox selected item. Here we data bind the ComboBox control with the items of a String array. We also add a selection changed event to the ComboBox control, so we can get the selected item when the user makes a selection on the ComboBox or change the existing selection.
The ItemsControl class’s ItemsSource property gets or sets an object source used to generate the content of the ItemsControl such as a ComboBox control. This property value is an Object instance that is the object that is used to generate the content of the ItemsControl. The default value of this property is null. So we can data bind the ComboBox control with a String array using this ItemsSource property.
The Selector class’s SelectionChanged event occurs when the currently selected item changes. And the Selector class’s SelectedItem property gets or sets the selected item. This property value is an Object instance that is the selected item. The default value of this property is null. So using the SelectionChanged event and SelectedItem property we can get the ComboBox selected item at runtime.
The following UWP app development tutorial code demonstrates how we can get the ComboBox selected item. Here we data bind the ComboBox control with the items of a String array. We also add a selection changed event to the ComboBox control, so we can get the selected item when the user makes a selection on the ComboBox or change the existing selection.
The ItemsControl class’s ItemsSource property gets or sets an object source used to generate the content of the ItemsControl such as a ComboBox control. This property value is an Object instance that is the object that is used to generate the content of the ItemsControl. The default value of this property is null. So we can data bind the ComboBox control with a String array using this ItemsSource property.
The Selector class’s SelectionChanged event occurs when the currently selected item changes. And the Selector class’s SelectedItem property gets or sets the selected item. This property value is an Object instance that is the selected item. The default value of this property is null. So using the SelectionChanged event and SelectedItem property we can get the ComboBox selected item at runtime.
MainPage.xaml
<Page
x:Class="UniversalAppTutorials.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:local="using:UniversalAppTutorials"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
mc:Ignorable="d">
<StackPanel
x:Name="stack_panel1"
Margin="50"
Orientation="Horizontal"
Background="AliceBlue"
Padding="50"
>
<ComboBox
x:Name="ComboBox1"
SelectionChanged="ComboBox1_SelectionChanged"
Margin="0,100,0,0"
/>
<TextBlock
x:Name="TextBlock1"
Foreground="Crimson"
FontFamily="MV Boli"
FontSize="25"
Text="Select a color from ComboBox."
TextWrapping="WrapWholeWords"
Margin="100,100,0,0"
/>
</StackPanel>
</Page>
MainPage.xaml.cs
using Windows.UI.Xaml.Controls;
namespace UniversalAppTutorials
{
public sealed partial class MainPage : Page
{
public MainPage()
{
this.InitializeComponent();
// Initialize a new string array
string[] colors = {
"Red",
"Green",
"Blue",
"Yellow",
"Snow",
"Gold",
"Forest Green",
"Medium Sea Green"
};
// Specify the ComboBox item source
ComboBox1.ItemsSource = colors;
}
private void ComboBox1_SelectionChanged(object sender, SelectionChangedEventArgs e)
{
// Get the instance of ComboBox
ComboBox comboBox = sender as ComboBox;
// Get the ComboBox selected item text
string selectedItems = comboBox.SelectedItem.ToString();
// Finally, display the ComboBox selected item text to text block
TextBlock1.Text = "Selected : " + selectedItems;
}
}
}
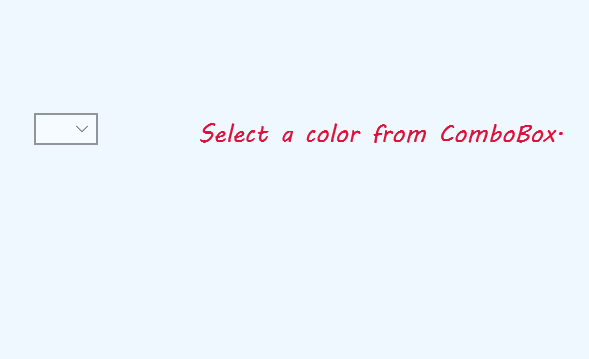
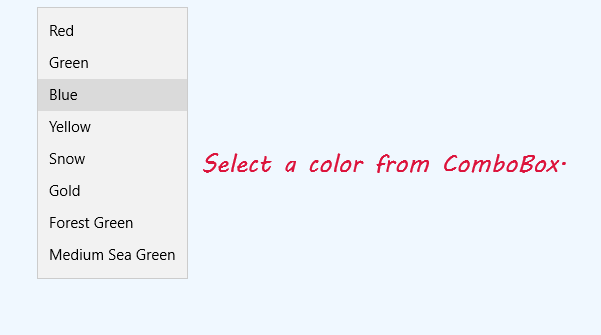
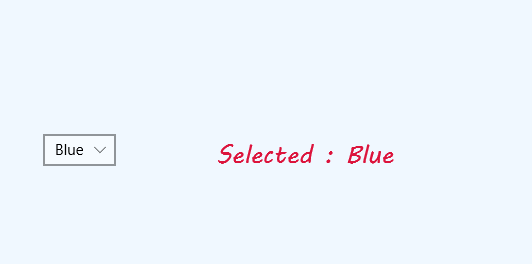
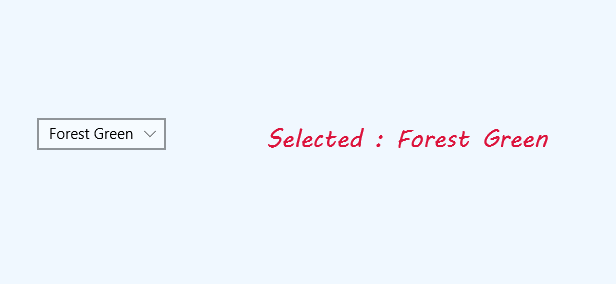