Create a ProgressBar In Code
ProgressBar is used to indicate the progress of an operation or long-running task, so the user can get a visual idea of how much time it will take to finish the job. We can simply add a ProgressBar widget to our XML layout file but when android developers want to put a ProgressBar in their app interface is a little bit harder. This android app development tutorial will guide you on how you can add a ProgressBar widget to your app rapidly in java code.
In the first step, we will create a ProgressBar in Java code. After that, we will set its layout parameters and define relative layout rules, and put it to relative layout on a specific location. The relative layout addView method allows us to add a dynamic view to it.
In the second step, we will show the operation progress in our newly added ProgressBar after a button click event. This is a determinate progress bar, so we can simply show the progress percentage in our widget. We also applied a color filter on our programmatically created ProgressBar widget.
The operation run on a background thread, so the app user interface is not blocked by the long-running task. We run an example task using runnable and handler in this tutorial. Button click starts the operation and the handler runs it periodically and completes it on time.
activity_main.xml
<RelativeLayout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/rl"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:padding="10dp"
tools:context=".MainActivity"
android:background="#d6e6de"
>
<Button
android:id="@+id/btn"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Create ProgressBar"
/>
<Button
android:id="@+id/btn_start"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Start Operation"
android:layout_toRightOf="@id/btn"
/>
<TextView
android:id="@+id/tv"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@id/btn_start"
/>
</RelativeLayout>
MainActivity.java
package com.cfsuman.me.androidcodesnippets;
import android.graphics.Color;
import android.graphics.PorterDuff;
import android.os.Bundle;
import android.app.Activity;
import android.view.View;
import android.widget.Button;
import android.widget.RelativeLayout;
import android.widget.TextView;
import android.widget.ProgressBar;
import android.os.Handler;
import android.widget.RelativeLayout.LayoutParams;
public class MainActivity extends Activity {
private int progressStatus = 0;
private Handler handler = new Handler();
ProgressBar pb;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Get the widgets reference from XML layout
final RelativeLayout rl = (RelativeLayout) findViewById(R.id.rl);
final Button btn = (Button) findViewById(R.id.btn);
final Button btn_start = (Button) findViewById(R.id.btn_start);
final TextView tv = (TextView) findViewById(R.id.tv);
btn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
//ProgressBar(Context context, AttributeSet attrs, int defStyleAttr)
// Initialize a new Progressbar instance
// Create a new progress bar programmatically
pb = new ProgressBar(getApplicationContext(), null, android.R.attr.progressBarStyleHorizontal);
// Create new layout parameters for progress bar
LayoutParams lp = new LayoutParams(
550, // Width in pixels
LayoutParams.WRAP_CONTENT // Height of progress bar
);
// Apply the layout parameters for progress bar
pb.setLayoutParams(lp);
// Get the progress bar layout parameters
LayoutParams params = (LayoutParams) pb.getLayoutParams();
// Set a layout position rule for progress bar
params.addRule(RelativeLayout.BELOW, tv.getId());
// Apply the layout rule for progress bar
pb.setLayoutParams(params);
// Set the progress bar color
pb.getProgressDrawable().setColorFilter(Color.BLUE, PorterDuff.Mode.SRC_IN);
// Finally, add the progress bar to layout
rl.addView(pb);
}
});
btn_start.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
// Set the progress status zero on each button click
progressStatus = 0;
// Start the lengthy operation in a background thread
new Thread(new Runnable() {
@Override
public void run() {
while(progressStatus < 100){
// Update the progress status
progressStatus +=1;
// Try to sleep the thread for 20 milliseconds
try{
Thread.sleep(20);
}catch(InterruptedException e){
e.printStackTrace();
}
// Update the progress bar
handler.post(new Runnable() {
@Override
public void run() {
pb.setProgress(progressStatus);
// Show the progress on TextView
tv.setText(progressStatus+"");
}
});
}
}
}).start(); // Start the operation
}
});
}
}
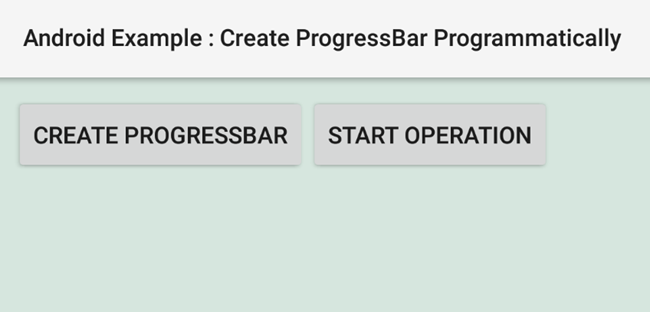
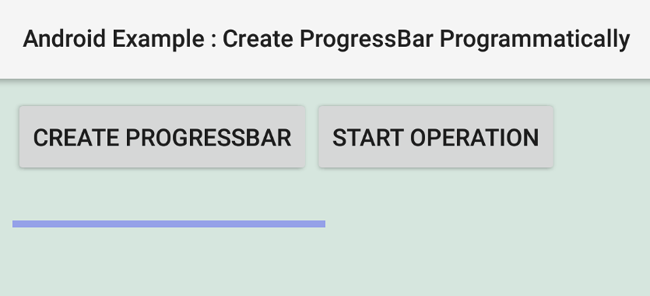
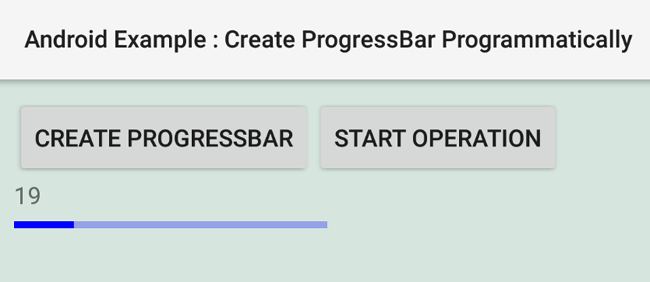
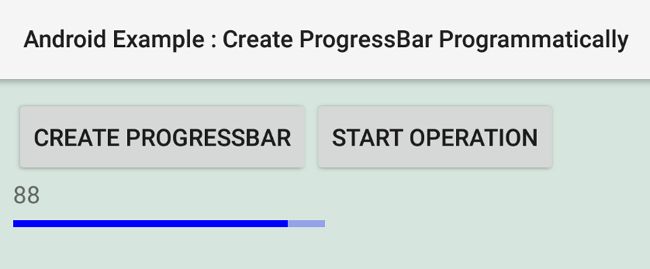