Format a String to a fixed length String
The String represents text as a sequence of UTF-16 code units. The
String is a sequential collection of characters that is used to represent
text. The String is a sequential collection of System.Char objects.
The following .net c# tutorial code demonstrates how we can format a String to a fixed-length String. In this .net c# tutorial code, we will format a small String instance to a fixed-length big String instance. Such as if the String length is five then we will format it to fifteen characters length. For the remaining extra length, we put specified characters.
The String PadLeft() method returns a new String of a specified length in which the beginning of the current String is padded with spaces or with a specified Unicode character.
The String PadLeft(int totalWidth, char paddingChar) method returns a new String that right-aligns the characters in the instance by padding them on the left with a specified Unicode character, for a specified total length. So, using this method overload, we can format a String instance to a fixed-length String. This method throws ArgumentOutOfRangeException if the ‘totalWidth’ is less than zero.
The following .net c# tutorial code demonstrates how we can format a String to a fixed-length String. In this .net c# tutorial code, we will format a small String instance to a fixed-length big String instance. Such as if the String length is five then we will format it to fifteen characters length. For the remaining extra length, we put specified characters.
The String PadLeft() method returns a new String of a specified length in which the beginning of the current String is padded with spaces or with a specified Unicode character.
The String PadLeft(int totalWidth, char paddingChar) method returns a new String that right-aligns the characters in the instance by padding them on the left with a specified Unicode character, for a specified total length. So, using this method overload, we can format a String instance to a fixed-length String. This method throws ArgumentOutOfRangeException if the ‘totalWidth’ is less than zero.
string-format-fixed-length.aspx
<%@ Page Language="C#" AutoEventWireup="true"%>
<!DOCTYPE html>
<script runat="server">
protected void Button1_Click(object sender, System.EventArgs e)
{
//this line create a string variable.
string stringValue = "red";
string stringValue2 = "green";
string stringValue3 = "yellow";
string stringValue4 = "crimson";
string stringValue5 = "indianred";
Label1.Text = "string value: " + stringValue.ToString();
Label1.Text += "<br />string value2: " + stringValue2.ToString();
Label1.Text += "<br />string value3: " + stringValue3.ToString();
Label1.Text += "<br />string value4: " + stringValue4.ToString();
Label1.Text += "<br />string value5: " + stringValue5.ToString();
Label1.Text += "<br /><br />formatted string<br /><br />";
//make string fixed length 25
Label1.Text += stringValue.PadLeft(25, '-');
Label1.Text += "<br />";
Label1.Text += stringValue2.PadLeft(25, '-');
Label1.Text += "<br />";
Label1.Text += stringValue3.PadLeft(25, '-');
Label1.Text += "<br />";
Label1.Text += stringValue4.PadLeft(25, '-');
Label1.Text += "<br />";
Label1.Text += stringValue5.PadLeft(25, '-');
//another technique to format string fixed length
//String.Format("color = |{0,25}|", stringValue);
}
</script>
<html xmlns="http://www.w3.org/1999/xhtml">
<head id="Head1" runat="server">
<title>c# example - string format fixed length</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<h2 style="color:MidnightBlue; font-style:italic;">
c# example - string format fixed length
</h2>
<hr width="550" align="left" color="Gainsboro" />
<asp:Label
ID="Label1"
runat="server"
Font-Size="Large"
>
</asp:Label>
<br /><br />
<asp:Button
ID="Button1"
runat="server"
Text="string format fixed length"
OnClick="Button1_Click"
Height="40"
Font-Bold="true"
/>
</div>
</form>
</body>
</html>
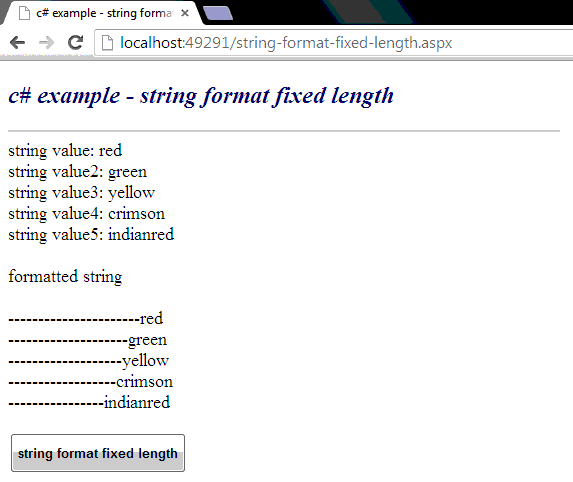