Check whether a String starts with a specific substring
The String represents text as a sequence of UTF-16 code units. The
String is a sequential collection of characters that is used to represent
text. The String is a sequential collection of System.Char objects.
The following .net c# tutorial code demonstrates how we can check whether a String instance starts with a specific substring. In this .net c# tutorial code we will check whether a String object starts with a specific substring or not by using the String StartsWith() method.
The String StartsWith(String) method overload determines whether the beginning of this String instance matches the specified String. So using this String StartsWith(string value) method overload .net developers can determine whether a String instance starts with a specific substring or not.
The String StartsWith(string value) method overload has a required parameter named value whose data type is a string. The value parameter is the String to compare. This method returns a Boolean value. It returns true if the value matches the beginning of this String otherwise the method returns false. The String StartsWith(string value) method overload throws ArgumentNullException if the provided value is null.
The following .net c# tutorial code demonstrates how we can check whether a String instance starts with a specific substring. In this .net c# tutorial code we will check whether a String object starts with a specific substring or not by using the String StartsWith() method.
The String StartsWith(String) method overload determines whether the beginning of this String instance matches the specified String. So using this String StartsWith(string value) method overload .net developers can determine whether a String instance starts with a specific substring or not.
The String StartsWith(string value) method overload has a required parameter named value whose data type is a string. The value parameter is the String to compare. This method returns a Boolean value. It returns true if the value matches the beginning of this String otherwise the method returns false. The String StartsWith(string value) method overload throws ArgumentNullException if the provided value is null.
string-starts-with.aspx
<%@ Page Language="C#" AutoEventWireup="true"%>
<!DOCTYPE html>
<script runat="server">
protected void Button1_Click(object sender, System.EventArgs e)
{
//this section create a string variable.
string stringPlants = "Boston Fern. Water Fern. European Flax. Wild Garli.";
Label1.Text = "string of plants..................<br />";
Label1.Text += stringPlants;
string stringStartsWith = "European";
string stringStartsWith2 = "Boston";
//this line check string starts with/ begins with 'European' or not
Boolean result = stringPlants.StartsWith(stringStartsWith);
//this line check string end with/ begins with 'Boston' or not
Boolean result2 = stringPlants.StartsWith(stringStartsWith2);
Label1.Text += "<br /><br /> string starts with [European]? " + result.ToString();
Label1.Text += "<br /><br /> string starts with [Boston]? " + result2.ToString();
}
</script>
<html xmlns="http://www.w3.org/1999/xhtml">
<head id="Head1" runat="server">
<title>c# example - string starts with</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<h2 style="color:MidnightBlue; font-style:italic;">
c# example - string starts with
</h2>
<hr width="550" align="left" color="Gainsboro" />
<asp:Label
ID="Label1"
runat="server"
Font-Size="Large"
>
</asp:Label>
<br /><br />
<asp:Button
ID="Button1"
runat="server"
Text="string starts with"
OnClick="Button1_Click"
Height="40"
Font-Bold="true"
/>
</div>
</form>
</body>
</html>
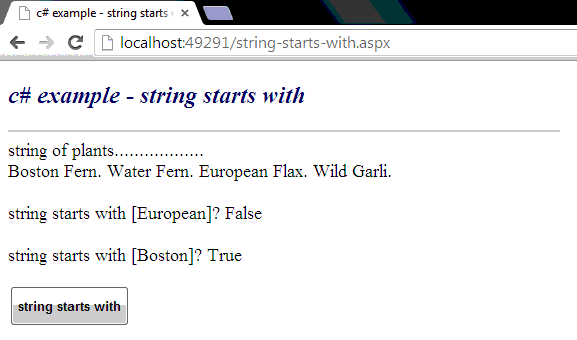