Find all elements from an Array that match conditions
The Array class provides methods for creating, manipulating, searching,
and sorting arrays. The Array class is not part of the System.Collections
namespaces. However, it is still considered a collection because it is based
on the IList interface. An element is a value in an Array. The length of an
Array is the total number of elements it can contain. The Array has a fixed
capacity.
The following .net c# tutorial code demonstrates how we can find all elements from an Array instance that match conditions. That means we will get all elements that satisfy the search criteria from an Array object. And also create an Array instance by using the returned elements. In this .net c# tutorial code, we will use the Array class FindAll() method to find all elements from an Array that match specified conditions.
The Array FindAll() method retrieves all the elements that match the conditions defined by the specified predicate. The FindAll<T> (T[] array, Predicate<T> match) method has two parameters those are array and match. The array parameter is the one-dimensional, zero-based Array to search. And the match parameter is the Predicate<T> that defines the conditions of the elements to search for.
The Array FindAll() method returns an Array containing all the elements that match the conditions defined by the specified predicate if found otherwise it returns an empty Array. The Array FindAll(array, match) method throws ArgumentNullException if the array is null or the match is null.
So finally, using the Array FindAll() method .net developers can find elements from an Array instance that match conditions. In this tutorial, we pass a condition that the element ends with a specified String by ignoring the case. We used the String class EndsWith() method to check the condition.
The following .net c# tutorial code demonstrates how we can find all elements from an Array instance that match conditions. That means we will get all elements that satisfy the search criteria from an Array object. And also create an Array instance by using the returned elements. In this .net c# tutorial code, we will use the Array class FindAll() method to find all elements from an Array that match specified conditions.
The Array FindAll() method retrieves all the elements that match the conditions defined by the specified predicate. The FindAll<T> (T[] array, Predicate<T> match) method has two parameters those are array and match. The array parameter is the one-dimensional, zero-based Array to search. And the match parameter is the Predicate<T> that defines the conditions of the elements to search for.
The Array FindAll() method returns an Array containing all the elements that match the conditions defined by the specified predicate if found otherwise it returns an empty Array. The Array FindAll(array, match) method throws ArgumentNullException if the array is null or the match is null.
So finally, using the Array FindAll() method .net developers can find elements from an Array instance that match conditions. In this tutorial, we pass a condition that the element ends with a specified String by ignoring the case. We used the String class EndsWith() method to check the condition.
array-findall.aspx
<%@ Page Language="C#" AutoEventWireup="true"%>
<!DOCTYPE html>
<script runat="server">
protected void Button1_Click(object sender, System.EventArgs e)
{
string[] birds = new string[]
{
"Snow Goose",
"Canada Goose",
"Muscovy Duck",
"Barnacle Goose",
"Fairy Penguin"
};
Label1.Text = "birds array.........<br />";
foreach (string s in birds)
{
Label1.Text += s + "<br />";
}
//find all elements which match search query
string[] endsWithGoose = Array.FindAll(birds, x => x.EndsWith("Goose", StringComparison.OrdinalIgnoreCase));
Label1.Text += "<br />all birds ends with [Goose] birds array.........<br />";
foreach (string s in endsWithGoose)
{
Label1.Text += s + "<br />";
}
}
</script>
<html xmlns="http://www.w3.org/1999/xhtml">
<head id="Head1" runat="server">
<title>c# example - array findall</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<h2 style="color:DarkBlue; font-style:italic;">
c# example - array findall
</h2>
<hr width="550" align="left" color="LightBlue" />
<asp:Label
ID="Label1"
runat="server"
Font-Size="X-Large"
>
</asp:Label>
<br />
<asp:Button
ID="Button1"
runat="server"
Text="test array findall"
OnClick="Button1_Click"
Height="40"
Font-Bold="true"
/>
</div>
</form>
</body>
</html>
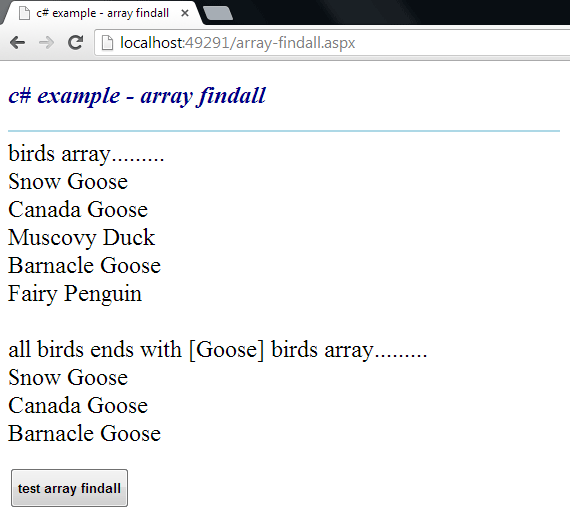