Convert double array to string
The following asp.net c# example code demonstrate us how can we convert
a double array to a string objectprogrammatically at run time in an
asp.net application. We can not directly convert a double array to string
object.
To convert, a double array to a string value, first we need to convert the double array to string array by convertingeach elements data type double to string. Then we can join the converted string array elements to create a string value.
.Net framework's array object work as like a collection, so we can apply Select method to an array. Using LinqSelect method we select all elements from the double array and convert each element value data type double to string.Finally, we convert the double array to string array by using Linq Select() method and ToArray() method.
Next, we can join each elements of double array to create a new string object. We can use a separator to join array elements.String Class String.Join() method concatenate the elements of a specified array or the members of a collection, using thespecified separator between each element or member.
String.Join(String, String[]) overloaded method concatenate all the elements of a string array, using the specified separatorbetween each element. Here we uses this overloaded method to convert the converted string array to string object. At last, we geta string object which value is converted from a double array and elements are separated by white space.
To convert, a double array to a string value, first we need to convert the double array to string array by convertingeach elements data type double to string. Then we can join the converted string array elements to create a string value.
.Net framework's array object work as like a collection, so we can apply Select method to an array. Using LinqSelect method we select all elements from the double array and convert each element value data type double to string.Finally, we convert the double array to string array by using Linq Select() method and ToArray() method.
Next, we can join each elements of double array to create a new string object. We can use a separator to join array elements.String Class String.Join() method concatenate the elements of a specified array or the members of a collection, using thespecified separator between each element or member.
String.Join(String, String[]) overloaded method concatenate all the elements of a string array, using the specified separatorbetween each element. Here we uses this overloaded method to convert the converted string array to string object. At last, we geta string object which value is converted from a double array and elements are separated by white space.
convert-double-array-to-string.aspx
<%@ Page Language="C#" AutoEventWireup="true"%>
<!DOCTYPE html>
<script runat="server">
protected void Button1_Click(object sender, System.EventArgs e)
{
double[] doublearray = new double[]
{
15.05,
10.23,
55.70,
29.89
};
Label1.Text = "double array.........<br />";
foreach (double d in doublearray)
{
Label1.Text += d.ToString() + "<br />";
}
string convertedstring = String.Join(" ", doublearray);
//another way tto convert double array items to string
//string convertedstring = String.Join(" ", doublearray.Select(x=>x.ToString()).ToArray());
Label1.Text += "<br />converted string: " + convertedstring;
}
</script>
<html xmlns="http://www.w3.org/1999/xhtml">
<head id="Head1" runat="server">
<title>c# example - convert double array to string</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<h2 style="color:DarkBlue; font-style:italic;">
c# example - convert double array to string
</h2>
<hr width="550" align="left" color="LightBlue" />
<asp:Label
ID="Label1"
runat="server"
Font-Size="Large"
>
</asp:Label>
<br /><br />
<asp:Button
ID="Button1"
runat="server"
Text="convert double array to string"
OnClick="Button1_Click"
Height="40"
Font-Bold="true"
/>
</div>
</form>
</body>
</html>
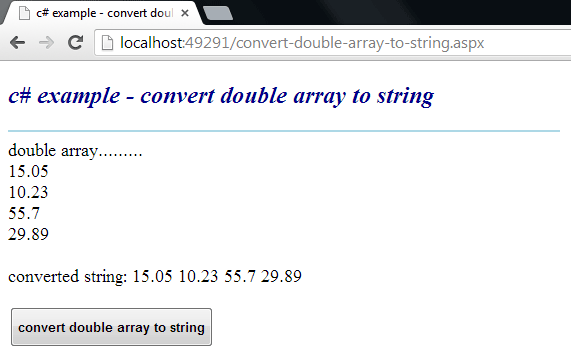