Convert a Decimal Array to a String Array
The Array class provides methods for creating, manipulating, searching,
and sorting arrays. The Array class is not part of the System.Collections
namespaces. However, it is still considered a collection because it is based
on the IList interface. An element is a value in an Array. The length of an
Array is the total number of elements it can contain. The Array has a fixed
capacity.
The following .net c# tutorial code demonstrates how we can convert a Decimal Array to a String Array. But there is no direct method in the Array class to convert a Decimal Array instance to a String Array instance. So, we have to do some tasks to get a String Array from Decimal Array elements.
The .net c# developers have to initialize an empty instance of a String Array at the beginning. While initializing the String Array instance, we will set the String Array size exactly the same as the Decimal Array size. Next, we have to loop through the Decimal Array elements. While iterating the Decimal Array elements, we also set the String Array’s elements value corresponding to the Decimal Array elements.
While setting the String Array’s elements value .net developers have to convert the Decimal value to a String instance. In this .net example code, we will use the Decimal class ToString() method to convert a Decimal instance to a String instance. So finally, we will get a String Array instance from the Decimal Array elements.
The Decimal class ToString() method converts the numeric value of this instance to its equivalent String representation. This method returns a string that represents the value of this instance. The Decimal class ToString() method formats a Decimal value in the default ("G", or general) format of the current culture.
The following .net c# tutorial code demonstrates how we can convert a Decimal Array to a String Array. But there is no direct method in the Array class to convert a Decimal Array instance to a String Array instance. So, we have to do some tasks to get a String Array from Decimal Array elements.
The .net c# developers have to initialize an empty instance of a String Array at the beginning. While initializing the String Array instance, we will set the String Array size exactly the same as the Decimal Array size. Next, we have to loop through the Decimal Array elements. While iterating the Decimal Array elements, we also set the String Array’s elements value corresponding to the Decimal Array elements.
While setting the String Array’s elements value .net developers have to convert the Decimal value to a String instance. In this .net example code, we will use the Decimal class ToString() method to convert a Decimal instance to a String instance. So finally, we will get a String Array instance from the Decimal Array elements.
The Decimal class ToString() method converts the numeric value of this instance to its equivalent String representation. This method returns a string that represents the value of this instance. The Decimal class ToString() method formats a Decimal value in the default ("G", or general) format of the current culture.
convert-decimal-array-to-string-array.aspx
<%@ Page Language="C#" AutoEventWireup="true"%>
<!DOCTYPE html>
<script runat="server">
protected void Button1_Click(object sender, System.EventArgs e)
{
decimal[] decimalarray = new decimal[]
{
5.28M,
2.93M,
11.5M,
2.82M,
9.5M
};
Label1.Text = "decimal array.........<br />";
foreach (decimal d in decimalarray)
{
Label1.Text += d.ToString() + "<br />";
}
string[] stringarray = new string[decimalarray.Length];
for (int i = 0; i < decimalarray.Length;i++ )
{
stringarray[i] = decimalarray[i].ToString();
//another way for conversion
//stringarray[i] = Convert.ToString(decimalarray[i]);
}
Label1.Text += "<br />converted string array.........<br />";
foreach (string s in stringarray)
{
Label1.Text += s + "<br />";
}
}
</script>
<html xmlns="http://www.w3.org/1999/xhtml">
<head id="Head1" runat="server">
<title>c# example - decimal array to string array</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<h2 style="color:DarkBlue; font-style:italic;">
c# example - convert decimal array to string array
</h2>
<hr width="550" align="left" color="LightBlue" />
<asp:Label
ID="Label1"
runat="server"
Font-Size="Large"
>
</asp:Label>
<br /><br />
<asp:Button
ID="Button1"
runat="server"
Text="convert decimal array to string array"
OnClick="Button1_Click"
Height="40"
Font-Bold="true"
/>
</div>
</form>
</body>
</html>
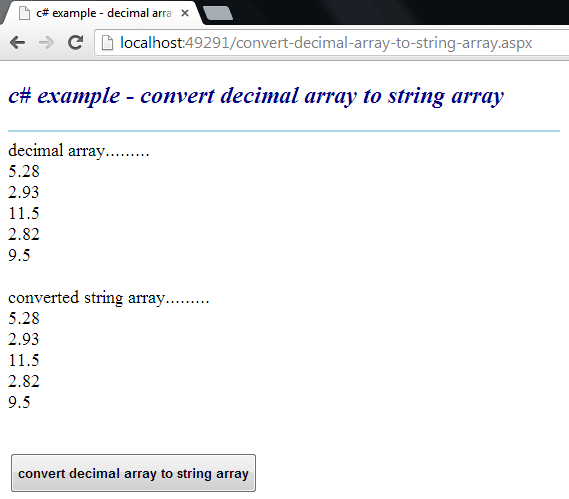