Create a new DataView
The DataView is a customized view of a DataTable for sorting, filtering,
searching, editing, and navigation. The DataView does not store data. When the
asp.net developer changes the DataView's data that will affect the DataTable.
But when they change the DataTable's data that will affect all associated
DataViews. The DataView is much like a database view.
The following asp.net c# tutorial code demonstrates how we can create a new DataView. Here we used the DataView class DataView(DataTable) constructor to create a new instance of the DataView object.
The DataView class DataView(DataTable) constructor initializes a new instance of the DataView class with the specified DataTable. The DataView (System.Data.DataTable? table) constructor has a parameter named table. The table parameter is a DataTable to add to the DataView.
The DataTable class represents one table of in-memory data. The DataTable is a central object in the ADO.NET library. The DataSet and DataView objects use DataTable.
So finally, the asp.net c# developers can create a new instance of a DataView object by using its various constructors. In the below example code, we used the DataView class DataView(DataTable) constructor to create a DataView from a specified DataTable instance.
The following asp.net c# tutorial code demonstrates how we can create a new DataView. Here we used the DataView class DataView(DataTable) constructor to create a new instance of the DataView object.
The DataView class DataView(DataTable) constructor initializes a new instance of the DataView class with the specified DataTable. The DataView (System.Data.DataTable? table) constructor has a parameter named table. The table parameter is a DataTable to add to the DataView.
The DataTable class represents one table of in-memory data. The DataTable is a central object in the ADO.NET library. The DataSet and DataView objects use DataTable.
So finally, the asp.net c# developers can create a new instance of a DataView object by using its various constructors. In the below example code, we used the DataView class DataView(DataTable) constructor to create a DataView from a specified DataTable instance.
HowToCreateADataView.aspx
<%@ Page Language="C#" AutoEventWireup="true" %>
<%@ Import Namespace="System.Data" %>
<!DOCTYPE html>
<script runat="server">
protected void Button1_Click(object sender, System.EventArgs e)
{
DataTable dt = new DataTable();
dt.TableName = "Books";
DataColumn dc1 = new DataColumn();
dc1.ColumnName = "BookID";
dc1.DataType = typeof(int);
dc1.AllowDBNull = false;
dc1.Unique = false;
DataColumn dc2 = new DataColumn();
dc2.ColumnName = "BookName";
dc2.DataType = typeof(string);
DataColumn dc3 = new DataColumn();
dc3.ColumnName = "Author";
dc3.DataType = typeof(string);
dt.Columns.AddRange(new DataColumn[] { dc1, dc2, dc3 });
dt.Rows.Add(new object[] { 1, "MySQL for Python", "Albert Lukaszewski, PhD" });
dt.Rows.Add(new object[] { 2, "Python 2.6 Graphics Cookbook", "Mike Ohlson de Fine " });
dt.Rows.Add(new object[] { 3, "Flash Game Development by Example", "Emanuele Feronato" });
dt.AcceptChanges();
GridView1.DataSource = dt.DefaultView;
GridView1.DataBind();
//this line create a new DataView
DataView dView = new DataView(dt);
dView.Sort = "BookName DESC";
Label1.Text = "Here we create a new DataView<br />" +
"and sorted it DESC by 'BookName' column";
GridView2.DataSource = dView;
GridView2.DataBind();
}
</script>
<html xmlns="http://www.w3.org/1999/xhtml">
<head id="Head1" runat="server">
<title>How to create a DataView in ado.net</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<h2 style="color:DarkBlue; font-style:italic;">
How to create a DataView in ado.net
</h2>
<hr width="450" align="left" color="CornFlowerBlue" />
<asp:GridView
ID="GridView1"
runat="server"
BorderColor="Snow"
ForeColor="Snow"
Width="525"
>
<HeaderStyle BackColor="PaleVioletRed" />
<RowStyle BackColor="SeaGreen" />
<AlternatingRowStyle BackColor="DarkGreen" />
</asp:GridView>
<br />
<asp:Label
ID="Label1"
runat="server"
Font-Size="Large"
ForeColor="DodgerBlue"
Font-Italic="true"
>
</asp:Label>
<br /><br />
<asp:GridView
ID="GridView2"
runat="server"
BorderColor="Snow"
ForeColor="Snow"
Width="525"
ShowHeaderWhenEmpty="true"
>
<HeaderStyle BackColor="PaleVioletRed" />
<RowStyle BackColor="SeaGreen" />
<AlternatingRowStyle BackColor="DarkGreen" />
</asp:GridView>
<br />
<asp:Button
ID="Button1"
runat="server"
OnClick="Button1_Click"
Text="Populate GridView"
Height="45"
Font-Bold="true"
ForeColor="DodgerBlue"
/>
</div>
</form>
</body>
</html>
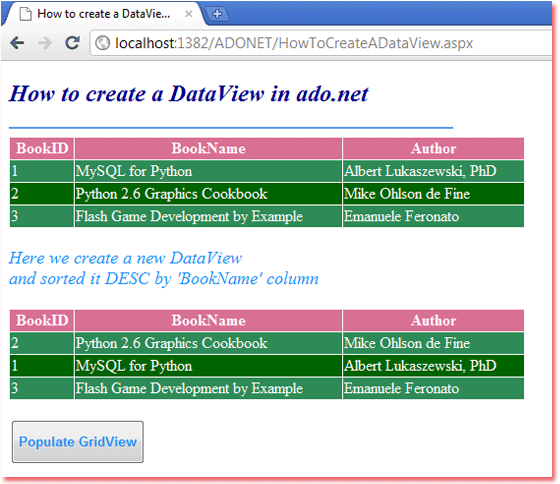