Insert a new row into the DataTable
The DataTable class represents one table of in-memory data. The
DataTable objects are conditionally case-sensitive. To create a DataTable
programmatically the asp.net developers must first define its schema by adding
DataColumn objects to the DataColumnCollection. To add rows to a DataTable,
the developers must use the NewRow() method to return a new DataRow object at
first. The DataTable also contains a collection of Constraint objects that can
be used to ensure the integrity of the data.
The following asp.net c# tutorial code demonstrates how we can insert a new row into a DataTable. So we have to insert a new DataRow object into the DataTable’s specified index position.
Here we used the DataTable class Rows property to get its rows collection as a DataRowCollection object. Then we used DataRowCollection’s InsertAt() method to insert a new DataRow object at a specified index position of the rows collections.
The DataTable class Rows property gets the collection of rows that belong to this table. The Rows property value is a DataRowCollection that contains DataRow objects. Each DataRow in the collection represents a row of data in the table. The DataRowCollection class represents a collection of rows for a DataTable.
The DataRowCollection InsertAt(DataRow, Int32) method inserts a new row into the collection at the specified location. The InsertAt(System.Data.DataRow row, int pos) method has two parameters. The row parameter is a DataRow to add. And the pos parameter is an Int32 which is the zero-based location in the collection where the asp.net web developers want to add the DataRow.
The InsertAt(System.Data.DataRow row, int pos) method throws IndexOutOfRangeException if the pos is less than 0. The location/index specified by InsertAt() method is reflected by the order of rows in the DataRowCollection only. If the value specified for the pos parameter of the InsertAt() method is greater than the number of rows in the collection then the new row is added to the end.
So finally, using the DataTable class Rows property the asp.net web developers can get the DataRowCollection object. And the DataRowCollection class InsertAt() method allows the asp.net web developers to insert a new row at the specified location of the row collection in a DataTable.
The following asp.net c# tutorial code demonstrates how we can insert a new row into a DataTable. So we have to insert a new DataRow object into the DataTable’s specified index position.
Here we used the DataTable class Rows property to get its rows collection as a DataRowCollection object. Then we used DataRowCollection’s InsertAt() method to insert a new DataRow object at a specified index position of the rows collections.
The DataTable class Rows property gets the collection of rows that belong to this table. The Rows property value is a DataRowCollection that contains DataRow objects. Each DataRow in the collection represents a row of data in the table. The DataRowCollection class represents a collection of rows for a DataTable.
The DataRowCollection InsertAt(DataRow, Int32) method inserts a new row into the collection at the specified location. The InsertAt(System.Data.DataRow row, int pos) method has two parameters. The row parameter is a DataRow to add. And the pos parameter is an Int32 which is the zero-based location in the collection where the asp.net web developers want to add the DataRow.
The InsertAt(System.Data.DataRow row, int pos) method throws IndexOutOfRangeException if the pos is less than 0. The location/index specified by InsertAt() method is reflected by the order of rows in the DataRowCollection only. If the value specified for the pos parameter of the InsertAt() method is greater than the number of rows in the collection then the new row is added to the end.
So finally, using the DataTable class Rows property the asp.net web developers can get the DataRowCollection object. And the DataRowCollection class InsertAt() method allows the asp.net web developers to insert a new row at the specified location of the row collection in a DataTable.
InsertDataRowIntoDataTable.aspx
<%@ Page Language="C#" AutoEventWireup="true" %>
<%@ Import Namespace="System.Data" %>
<!DOCTYPE html>
<script runat="server">
void Button1_Click(object sender, System.EventArgs e)
{
DataTable dt = new DataTable();
dt.TableName = "Books";
DataColumn dc1 = new DataColumn();
dc1.ColumnName = "BookID";
dc1.DataType = typeof(int);
dc1.AllowDBNull = false;
dc1.Unique = true;
DataColumn dc2 = new DataColumn();
dc2.ColumnName = "BookName";
dc2.DataType = typeof(string);
DataColumn dc3 = new DataColumn();
dc3.ColumnName = "Author";
dc3.DataType = typeof(string);
dt.Columns.AddRange(new DataColumn[] { dc1,dc2,dc3 });
dt.Rows.Add(new object[] { 1, "Learning Flash Media Server 3", "William Sanders" });
dt.Rows.Add(new object[] { 2, "Essential ActionScript 3.0", "Colin Moock" });
dt.Rows.Add(new object[] { 3, "Learning Flash Media Server 2", "William Sanders" });
GridView1.DataSource = dt;
GridView1.DataBind();
DataRow dr = dt.NewRow();
dr["BookID"] = 4;
dr["BookName"] = "Introduction to Flex 2";
dr["Author"] = "Roger Braunstein";
//this line add the new DataRow into DataTable at the specified location
dt.Rows.InsertAt(dr,1);
Label1.Text = "After inserting the new DataRow at location 1";
GridView2.DataSource = dt;
GridView2.DataBind();
}
</script>
<html xmlns="http://www.w3.org/1999/xhtml">
<head id="Head1" runat="server">
<title>How to insert a new DataRow into DataTable at the specified location in ado.net</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<h2 style="color:DarkBlue; font-style:italic;">
How to insert a new DataRow into
<br /> DataTable at the specified location in ado.net
</h2>
<hr width="500" align="left" color="CornFlowerBlue" />
<asp:GridView
ID="GridView1"
runat="server"
BorderColor="Snow"
ForeColor="Snow"
Width="550"
>
<HeaderStyle BackColor="MediumVioletRed" Height="35" />
<RowStyle BackColor="Violet" />
<AlternatingRowStyle BackColor="Thistle" />
</asp:GridView>
<br />
<asp:Label
ID="Label1"
runat="server"
Font-Size="Medium"
ForeColor="SeaGreen"
>
</asp:Label>
<br /><br />
<asp:GridView
ID="GridView2"
runat="server"
BorderColor="Snow"
ForeColor="Snow"
Width="550"
>
<HeaderStyle BackColor="MediumVioletRed" Height="35" />
<RowStyle BackColor="Violet" />
<AlternatingRowStyle BackColor="Thistle" />
</asp:GridView>
<asp:Button
ID="Button1"
runat="server"
OnClick="Button1_Click"
Text="Populate GridView"
Height="45"
Font-Bold="true"
ForeColor="DarkBlue"
/>
</div>
</form>
</body>
</html>
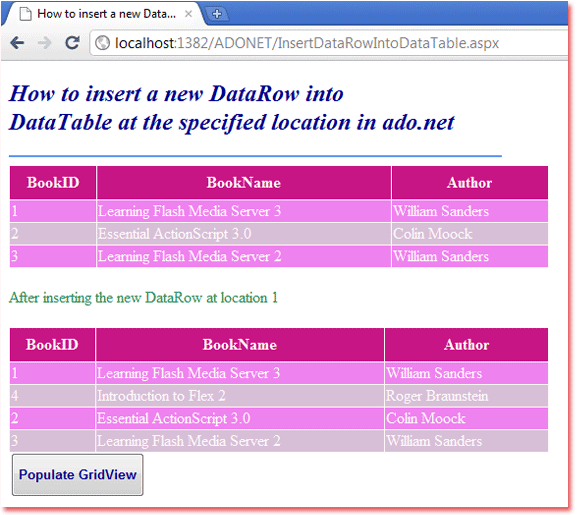