Delete a row from the DataTable
The DataTable class represents one table of in-memory data. The
DataTable objects are conditionally case-sensitive. To create a DataTable
programmatically the asp.net developers must first define its schema by adding
DataColumn objects to the DataColumnCollection. To add rows to a DataTable,
the developers must use the NewRow() method to return a new DataRow object at
first. The DataTable also contains a collection of Constraint objects that can
be used to ensure the integrity of the data.
The following asp.net c# tutorial code demonstrates how we can delete a row from a DataTable. So we will remove a DataRow object from a DataTable instance at the specified index position. In this example code, we will remove a row by using its index value from a DataTable object.
Here we used the DataTable class Rows property to get its rows collection as a DataRowCollection object. Then we used DataRowCollection’s RemoveAt() method to remove a DataRow object at the specified index position from the rows collections.
The DataTable class Rows property gets the collection of rows that belong to this table. The Rows property value is a DataRowCollection that contains DataRow objects. Each DataRow in the collection represents a row of data in the table. The DataRowCollection class represents a collection of rows for a DataTable.
The DataRowCollection RemoveAt(Int32) method removes the row at the specified index from the collection. The RemoveAt(int index) method has a parameter named index. The index parameter is an Int32 which is the index of the row to remove.
When a row is removed from a DataTable, all data in that row is lost. The asp.net web developers can also call the Delete() method of the DataRow class to just mark a row for removal. Calling the RemoveAt() method is the same as calling the Delete() method and then calling AcceptChanges. The asp.net web developers also can use the Clear() method to remove all members of the rows collection at one time.
So finally, using the DataTable class Rows property the asp.net web developers can get the DataRowCollection object. And the DataRowCollection class RemoveAt() method allows the asp.net web developers to remove a row from the row collection in a DataTable at the specified index position.
The following asp.net c# tutorial code demonstrates how we can delete a row from a DataTable. So we will remove a DataRow object from a DataTable instance at the specified index position. In this example code, we will remove a row by using its index value from a DataTable object.
Here we used the DataTable class Rows property to get its rows collection as a DataRowCollection object. Then we used DataRowCollection’s RemoveAt() method to remove a DataRow object at the specified index position from the rows collections.
The DataTable class Rows property gets the collection of rows that belong to this table. The Rows property value is a DataRowCollection that contains DataRow objects. Each DataRow in the collection represents a row of data in the table. The DataRowCollection class represents a collection of rows for a DataTable.
The DataRowCollection RemoveAt(Int32) method removes the row at the specified index from the collection. The RemoveAt(int index) method has a parameter named index. The index parameter is an Int32 which is the index of the row to remove.
When a row is removed from a DataTable, all data in that row is lost. The asp.net web developers can also call the Delete() method of the DataRow class to just mark a row for removal. Calling the RemoveAt() method is the same as calling the Delete() method and then calling AcceptChanges. The asp.net web developers also can use the Clear() method to remove all members of the rows collection at one time.
So finally, using the DataTable class Rows property the asp.net web developers can get the DataRowCollection object. And the DataRowCollection class RemoveAt() method allows the asp.net web developers to remove a row from the row collection in a DataTable at the specified index position.
RemoveDataRowFromDataTable.aspx
<%@ Page Language="C#" AutoEventWireup="true" %>
<%@ Import Namespace="System.Data" %>
<!DOCTYPE html>
<script runat="server">
void Button1_Click(object sender, System.EventArgs e)
{
DataTable dt = new DataTable();
dt.TableName = "Books";
DataColumn dc1 = new DataColumn();
dc1.ColumnName = "BookID";
dc1.DataType = typeof(int);
dc1.AllowDBNull = false;
dc1.Unique = true;
DataColumn dc2 = new DataColumn();
dc2.ColumnName = "BookName";
dc2.DataType = typeof(string);
DataColumn dc3 = new DataColumn();
dc3.ColumnName = "Author";
dc3.DataType = typeof(string);
dt.Columns.AddRange(new DataColumn[] { dc1,dc2,dc3 });
dt.Rows.Add(new object[] { 1, "Flash 8 Cookbook", "Joey Lott" });
dt.Rows.Add(new object[] { 2, "Flash 8: The Missing Manual", "E. A. Vander Veer" });
dt.Rows.Add(new object[] { 3, "Flash Out of the Box", "Robert Hoekman, Jr." });
GridView1.DataSource = dt;
GridView1.DataBind();
//this line remove the DataRow from DataTable which index is 2
dt.Rows.RemoveAt(2);
Label1.Text = "After delete the DataRow which index is 2";
GridView2.DataSource = dt;
GridView2.DataBind();
}
</script>
<html xmlns="http://www.w3.org/1999/xhtml">
<head id="Head1" runat="server">
<title>How to remove (delete) a DataRow at the specified index from DataTable in ado.net</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<h2 style="color:DarkBlue; font-style:italic;">
How to remove (delete) a DataRow
<br /> at the specified index from DataTable in ado.net
</h2>
<hr width="500" align="left" color="CornFlowerBlue" />
<asp:GridView
ID="GridView1"
runat="server"
BorderColor="Snow"
ForeColor="Snow"
Width="550"
>
<HeaderStyle BackColor="OliveDrab" Height="35" />
<RowStyle BackColor="DarkOrchid" />
<AlternatingRowStyle BackColor="MediumOrchid" />
</asp:GridView>
<br />
<asp:Label
ID="Label1"
runat="server"
Font-Size="Medium"
ForeColor="SeaGreen"
>
</asp:Label>
<br /><br />
<asp:GridView
ID="GridView2"
runat="server"
BorderColor="Snow"
ForeColor="Snow"
Width="550"
>
<HeaderStyle BackColor="OliveDrab" Height="35" />
<RowStyle BackColor="DarkOrchid" />
<AlternatingRowStyle BackColor="MediumOrchid" />
</asp:GridView>
<asp:Button
ID="Button1"
runat="server"
OnClick="Button1_Click"
Text="Populate GridView"
Height="45"
Font-Bold="true"
ForeColor="DarkBlue"
/>
</div>
</form>
</body>
</html>
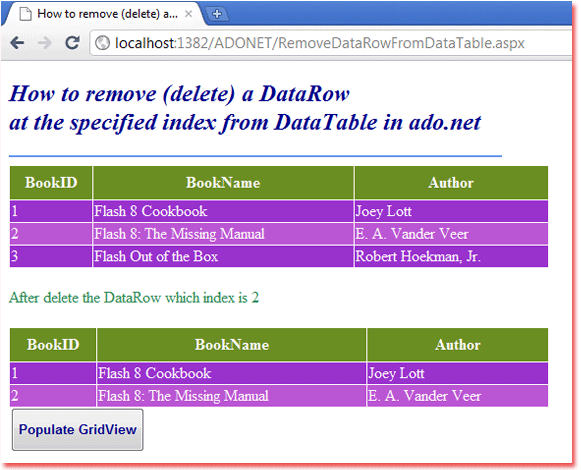