Add an item to BulletedList programmatically
BulletedList is an ASP.NET list web server control. ASP.NET developers
use BulletedList server control to create a list of items that are formatted
with bullets. The BulletedList Items property gets the collection of items in
the BulletedList control. Items collection contains all ListItem objects. A
ListItem object represents a single item in BulletedList control.
ListItem object has three properties that are Text, Value, and Selected. Text property text is displayed in BulletedList control and Value property text is associated with ListItem and is hidden in the browser. The Selected property indicates whether ListItem is selected or not.
We can add ListItem objects of BulletedList server control at design time. We also can populate BulletedList by data binding with data source control. Even we can add items to BulletedList control programmatically at run time.
To add an item programmatically in BulletedList control, first, we need to create a ListItem object. Next, we need to add the ListItem object in the Items collection of BulletedList control. Now BulletedList will display the newly added item.
The following c# example code demonstrates to us how can we add an item in BulletedList server control programmatically (dynamically) at run time in ASP.NET.
ListItem object has three properties that are Text, Value, and Selected. Text property text is displayed in BulletedList control and Value property text is associated with ListItem and is hidden in the browser. The Selected property indicates whether ListItem is selected or not.
We can add ListItem objects of BulletedList server control at design time. We also can populate BulletedList by data binding with data source control. Even we can add items to BulletedList control programmatically at run time.
To add an item programmatically in BulletedList control, first, we need to create a ListItem object. Next, we need to add the ListItem object in the Items collection of BulletedList control. Now BulletedList will display the newly added item.
The following c# example code demonstrates to us how can we add an item in BulletedList server control programmatically (dynamically) at run time in ASP.NET.
BulletedListAddItem.aspx
<%@ Page Language="C#" %>
<!DOCTYPE html>
<script runat="server">
protected void Button1_Click(object sender, System.EventArgs e)
{
ListItem li = new ListItem();
li.Text = TextBox1.Text.ToString();
BulletedList1.Items.Add(li);
Label1.Text = "ListItem added in BulletedList: " + li.Text;
}
</script>
<html xmlns="http://www.w3.org/1999/xhtml">
<head id="Head1" runat="server">
<title>How to add list item in BulletedList programmatically</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<h2 style="color:Red">BulletedList example: Add List Item</h2>
<asp:Label
ID="Label1"
runat="server"
Font-Bold="true"
ForeColor="SeaGreen"
Font-Size="Large"
>
</asp:Label>
<br /><br />
<asp:Label
ID="Label2"
runat="server"
Text="Color List"
Font-Bold="true"
ForeColor="DodgerBlue"
>
</asp:Label>
<br />
<asp:BulletedList
ID="BulletedList1"
runat="server"
BackColor="DodgerBlue"
ForeColor="Snow"
Width="250"
>
<asp:ListItem>Magenta</asp:ListItem>
<asp:ListItem>MediumBlue</asp:ListItem>
<asp:ListItem>Orchid</asp:ListItem>
<asp:ListItem>PaleGoldenRod</asp:ListItem>
<asp:ListItem>Maroon</asp:ListItem>
</asp:BulletedList>
<br />
<asp:Label
ID="Label3"
runat="server"
ForeColor="DodgerBlue"
Text="Item Text"
>
</asp:Label>
<asp:TextBox
ID="TextBox1"
runat="server"
BackColor="DodgerBlue"
ForeColor="Snow"
>
</asp:TextBox>
<br />
<asp:Button
ID="Button1"
runat="server"
Text="Add List Item"
Font-Bold="true"
ForeColor="DodgerBlue"
OnClick="Button1_Click"
/>
</div>
</form>
</body>
</html>
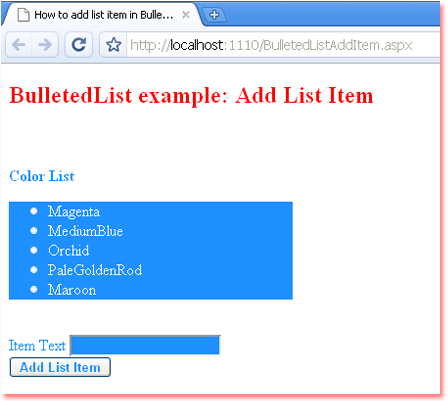
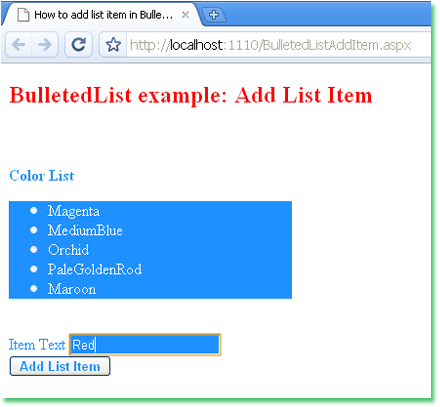
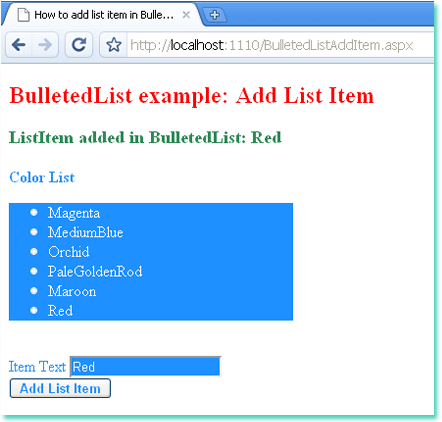