Change Label width programmatically
The Label class Represents a label control, which displays text on a Web
page. The asp.net developers can use the Label control to display text in a
set location on the web page. Unlike static text, asp.net c# developers can
customize the displayed text through the Text property.
The following asp.net c# tutorial code demonstrates how we can programmatically change a Label control’s width. Here we used the Label web server control’s Width property to change its width programmatically.
The Label control Width property gets or sets the width of the Web server control. The Width property value is a Unit that represents the width of the Label control. The default value of this property is Empty. The Unit structure represents a length measurement.
The Label control’s Width property throws ArgumentException if the width of the Web server control was set to a negative value. So finally, the asp.net c# developers can use the Width property to change the width of the Label Web server control programmatically.
The following asp.net c# tutorial code demonstrates how we can programmatically change a Label control’s width. Here we used the Label web server control’s Width property to change its width programmatically.
The Label control Width property gets or sets the width of the Web server control. The Width property value is a Unit that represents the width of the Label control. The default value of this property is Empty. The Unit structure represents a length measurement.
The Label control’s Width property throws ArgumentException if the width of the Web server control was set to a negative value. So finally, the asp.net c# developers can use the Width property to change the width of the Label Web server control programmatically.
LabelWidth.aspx
<%@ Page Language="C#" %>
<!DOCTYPE html>
<script runat="server">
protected void Button1_Click(object sender, System.EventArgs e)
{
Label1.Width = 75;
}
protected void Button2_Click(object sender, System.EventArgs e)
{
Label1.Width = 250;
}
</script>
<html xmlns="http://www.w3.org/1999/xhtml">
<head id="Head1" runat="server">
<title>How to set, change Label width programmatically</title>
</head>
<body>
<form id="form1" runat="server">
<div>
<h2 style="color:Red">Label Example: Width</h2>
<asp:Label
ID="Label1"
runat="server"
Text="Change Label Width."
BackColor="SaddleBrown"
ForeColor="Snow"
BorderWidth="3"
BorderColor="DodgerBlue"
Font-Size="Large"
>
</asp:Label>
<br /><br />
<asp:Button
ID="Button1"
runat="server"
ForeColor="SaddleBrown"
Text="Label Width 75"
OnClick="Button1_Click"
Font-Bold="true"
/>
<asp:Button
ID="Button2"
runat="server"
Font-Bold="true"
ForeColor="SaddleBrown"
Text="Label Width 250"
OnClick="Button2_Click"
/>
</div>
</form>
</body>
</html>
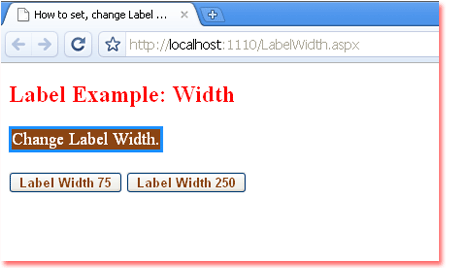
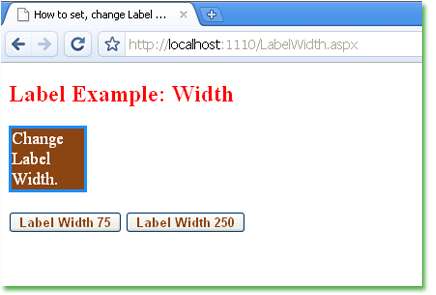
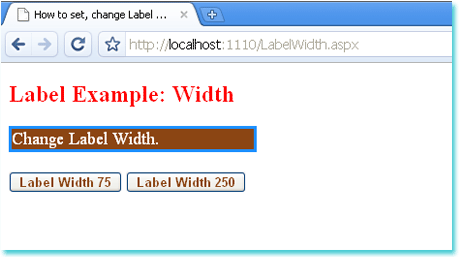