Introduction
In Adobe ColdFusion, working with lists is a common task, especially when developers need to manage multiple items stored in a single variable. When handling lists, case sensitivity often becomes an obstacle, as ColdFusion's default behavior is case-sensitive in its searches. To address this issue, ColdFusion offers a built-in function called ListFindNoCase
, which allows developers to search for elements within a list without worrying about letter case differences. This tutorial demonstrates how to use the ListFindNoCase
function by implementing a simple example where we locate specific color names within a list.
Setting Up the List and Displaying It
In this example, we begin by defining a variable, ColorList
, which contains a series of color names separated by commas. These colors are listed in mixed casing to simulate real-world data, where inconsistencies in capitalization may arise. Displaying the list at the start helps users understand the elements stored within ColorList
before the search process begins. The colors in this list include "DarkViolet," "DeepPink," "DarkViolet," and "DeepSkyBlue," with some colors intentionally repeated to illustrate how ListFindNoCase
can handle duplicate elements.
The ListFindNoCase
Function Overview
The core function of this tutorial is ListFindNoCase
, which is used here to search for various color names within ColorList
. This function takes three main arguments:
- The list to search within (in this case,
ColorList
). - The item or string to look for within the list.
- The delimiter used in the list (commas, in this case).
When executed, ListFindNoCase
searches for the specified item in a case-insensitive manner, meaning "DarkViolet," "darkviolet," and "DARKVIOLET" would all match the same color. The function returns the position of the first occurrence of the specified element, with 1 indicating the first item in the list, 2 the second, and so on. If the item is not found, ListFindNoCase
returns 0.
Example of Case-Insensitive Searches with ListFindNoCase
To demonstrate ListFindNoCase
in action, we search for different color names in both matching and non-matching cases:
- The first search attempts to find "DarkViolet," matching the case exactly as it appears in
ColorList
. The result shows the first position where "DarkViolet" is found. - The second search attempts to find "darkViolet," with different casing. Thanks to the case-insensitive nature of
ListFindNoCase
, it still successfully locates the color and returns the position. - We then search for "Red," a color not present in
ColorList
. As expected,ListFindNoCase
returns 0, indicating that "Red" is not found. - Finally, we search for "deepskyblue," again in a different case than it appears in the list. The function identifies the color correctly, demonstrating the flexibility of case-insensitive matching.
Displaying the Results in a Table Format
For a clear presentation of results, this example outputs the search results in an HTML table. Each row represents a different search query, displaying both the function call and its output. The table’s colors are customized to enhance readability and make the results visually appealing. This organized structure helps users quickly understand how ListFindNoCase
works with various cases and input values.
Conclusion
The ListFindNoCase
function in ColdFusion provides an efficient solution for locating elements within lists without regard to letter casing. This is particularly useful for developers who handle user-generated data or deal with data inconsistencies. By following this example, developers can effectively incorporate ListFindNoCase
into their own ColdFusion applications, ensuring that searches within lists are case-insensitive and thus more user-friendly. This tutorial demonstrates the utility of ListFindNoCase
in managing data smoothly, even when inputs may not always match in case.
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>ListFindNoCase function example: how to find List element (case insensitive)</title>
</head>
<body>
<h2 style="color:hotpink">ListFindNoCase Function Example: Case-insensitive</h2>
<cfset ColorList="DarkViolet,DeepPink,DarkViolet,DeepSkyBlue">
<cfoutput><b>ColorList: #ColorList#</b></cfoutput>
<br /><br />
<table style="color:LightGreen; background:Green;" border="1" bordercolor="DarkGreen">
<tr>
<td style="font-weight:bold">ListFindNoCase Case-insensitive</td>
<td style="font-weight:bold">Output</td>
</tr>
<tr>
<td>#ListFindNoCase(ColorList,"DarkViolet",",")#</td>
<td><cfoutput>#ListFindNoCase(ColorList,"DarkViolet",",")#</cfoutput></td>
</tr>
<tr>
<td>#ListFindNoCase(ColorList,"darkViolet",",")#</td>
<td><cfoutput>#ListFindNoCase(ColorList,"darkViolet",",")#</cfoutput></td>
</tr>
<tr>
<td>#ListFindNoCase(ColorList,"Red",",")#</td>
<td><cfoutput>#ListFindNoCase(ColorList,"Red",",")#</cfoutput></td>
</tr>
<tr>
<td>#ListFindNoCase(ColorList,"deepskyblue",",")#</td>
<td><cfoutput>#ListFindNoCase(ColorList,"deepskyblue",",")#</cfoutput></td>
</tr>
</table>
</body>
</html>
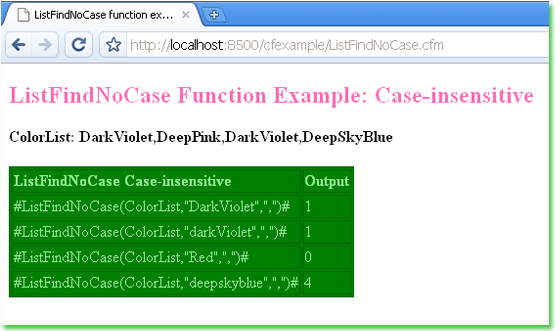
- ListDeleteAt() - delete List element at specific position
- ListFind() - find List element (case sensitive)
- ListFirst() - get the first element of a List
- ListGetAt() - get List element at a specified position
- ListLast() - get the last element of a list
- ListLen() - get total number of elements in a List