Introduction
In ColdFusion, working with dates is a frequent task in web development. One of the common requirements is calculating the difference between two date-time objects. ColdFusion provides a built-in function, DateDiff()
, which simplifies this process by returning the difference between two dates in a specified date part (e.g., years, months, or days). This article breaks down an example where we use the DateDiff()
function to compare two dates and display their differences in various units, including years, quarters, months, and days.
The purpose of this tutorial is to demonstrate how to work with the DateDiff()
function in ColdFusion and explore how it can be used to calculate differences between date objects efficiently. We’ll cover how the function works, and how the results are presented in an HTML table format.
Setting Date Variables
The first part of the code involves setting two date variables, MyDate
and MyAnotherDate
. These variables store two different dates: December 8, 2006, and December 10, 2007, respectively. ColdFusion allows easy assignment of date strings using the <cfset>
tag. The dates will later be compared using the DateDiff()
function to calculate differences in years, quarters, months, and days.
Outputting the Date Values
Before we calculate the differences, we output the original dates using the <cfoutput>
tag. This tag allows dynamic content (like ColdFusion variables) to be embedded directly in the HTML output. The values of MyDate
and MyAnotherDate
are printed in bold, making it clear which dates are being compared.
Calculating Date Differences Using DateDiff()
The core of the tutorial lies in calculating date differences using the DateDiff()
function. The function takes three arguments:
- DatePart: The unit of time to measure the difference in (e.g., years, months, days).
- Start Date: The earlier date (in this case,
MyDate
). - End Date: The later date (in this case,
MyAnotherDate
).
In this example, we use four different date parts:
"yyyy"
for years"q"
for quarters"m"
for months"d"
for days
Each calculation is performed and displayed in an HTML table format, making the differences between the two dates visually clear.
Displaying the Results in an HTML Table
The results of the DateDiff()
function are presented in a well-structured HTML table. The table has three columns:
- DateDiff: Displays the ColdFusion function used for each comparison (e.g.,
DateDiff("yyyy", MyDate, MyAnotherDate)
). - DatePart: Describes the unit of time being measured (e.g., years, quarters, months, days).
- Output: Displays the actual numerical difference between the two dates for the respective date part.
This approach allows the results to be clearly viewed side-by-side, making it easy for the user to understand the relationship between the two dates in different time units.
Styling the Output
To make the output more visually appealing, some basic CSS styling is applied to the table. The rows have a green background with light green text, while the headers are bold and emphasize the table structure. This simple styling enhances readability and makes the output more engaging for the user.
Summary
The ColdFusion DateDiff()
function is a powerful tool for comparing two date-time objects and obtaining the difference in various units of time. This tutorial demonstrates how to use this function to measure differences in years, quarters, months, and days, and display the results in a well-organized HTML table. By setting date variables, calculating the differences, and formatting the output, we achieve a clear and informative display of the date comparisons.
This example provides a foundation for using date calculations in ColdFusion. Whether you need to track time elapsed between events or create more complex time-based applications, the DateDiff()
function offers flexibility and ease of use.
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>DateDiff function example: how to get difference between two date time object</title>
</head>
<body>
<h2 style="color:hotpink">DateDiff Function Example</h2>
<cfset MyDate="8-Dec-2006">
<cfset MyAnotherDate="10-Dec-2007">
<cfoutput>
<b>
MyDate: #MyDate#
<br />
MyAnotherDate: #MyAnotherDate#
<br /><br />
</b>
</cfoutput>
<table style="color:LightGreen; background:Green;" border="1" bordercolor="DarkGreen">
<tr>
<td style="font-weight:bold">DateDiff</td>
<td style="font-weight:bold">DatePart</td>
<td style="font-weight:bold">Output</td>
</tr>
<tr>
<td>#DateDiff("yyyy",MyDate,MyAnotherDate)#</td>
<td>Years</td>
<td><cfoutput>#DateDiff("yyyy",MyDate,MyAnotherDate)#</cfoutput></td>
</tr>
<tr>
<td>#DateDiff("q",MyDate,MyAnotherDate)#</td>
<td>Quarters</td>
<td><cfoutput>#DateDiff("q",MyDate,MyAnotherDate)#</cfoutput></td>
</tr>
<tr>
<td>#DateDiff("m",MyDate,MyAnotherDate)#</td>
<td>Months</td>
<td><cfoutput>#DateDiff("m",MyDate,MyAnotherDate)#</cfoutput></td>
</tr>
<tr>
<td>#DateDiff("d",MyDate,MyAnotherDate)#</td>
<td>Days</td>
<td><cfoutput>#DateDiff("d",MyDate,MyAnotherDate)#</cfoutput></td>
</tr>
</table>
</body>
</html>
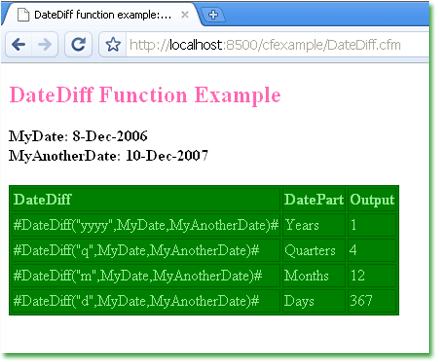
- CreateDate() - create a date object
- DateAdd() - add year, quarter, month, week, day, hour, minute, second to a date
- DateCompare() - compare two date time object
- DateFormat() - format a date value using U.S. date format
- DatePart() - get a part from a date value
- Quarter() - get quarter number from a date object